The box model
In the default box model, when you set the width or height of an element, you are specifying the width or
height of its content; any padding, border, and margins are then added to that width.
We can adjust the box model behavior with its box sizing property.
- box-sizing: content-box (by default) any height or width you specify only sets the size of the content box.
- box-sizing: border-box the height and width properties set the combined size of the content, padding, and border.
Top
<-----------Left----------->
Margin
Border
Padding
Content
<-----------Width----------->
<-----------Height----------->
<-----------Right----------->
Bottom
CSS layouts
Float
Floats are the oldest method for laying out a web.
A float pulls an element (often an image) to one side of its
container, allowing the document flow to wrap around it.
If you float multiple elements in the same direction, they will
stack alongside one anothe
Floats are still the only way to move an image to the side of the
page and allow text to wrap around it.
HTML
< p >
Lorem, ipsum dolor sit amet consectetur adipisicing elit. Accusamus rem ad aliquid vero, cumque eligendi quasi impedit ipsam quos voluptas aperiam reiciendis placeat, cum recusandae sapiente. Ullam iure laudantium modi!
</p >
CSS
float: left;
}
Result
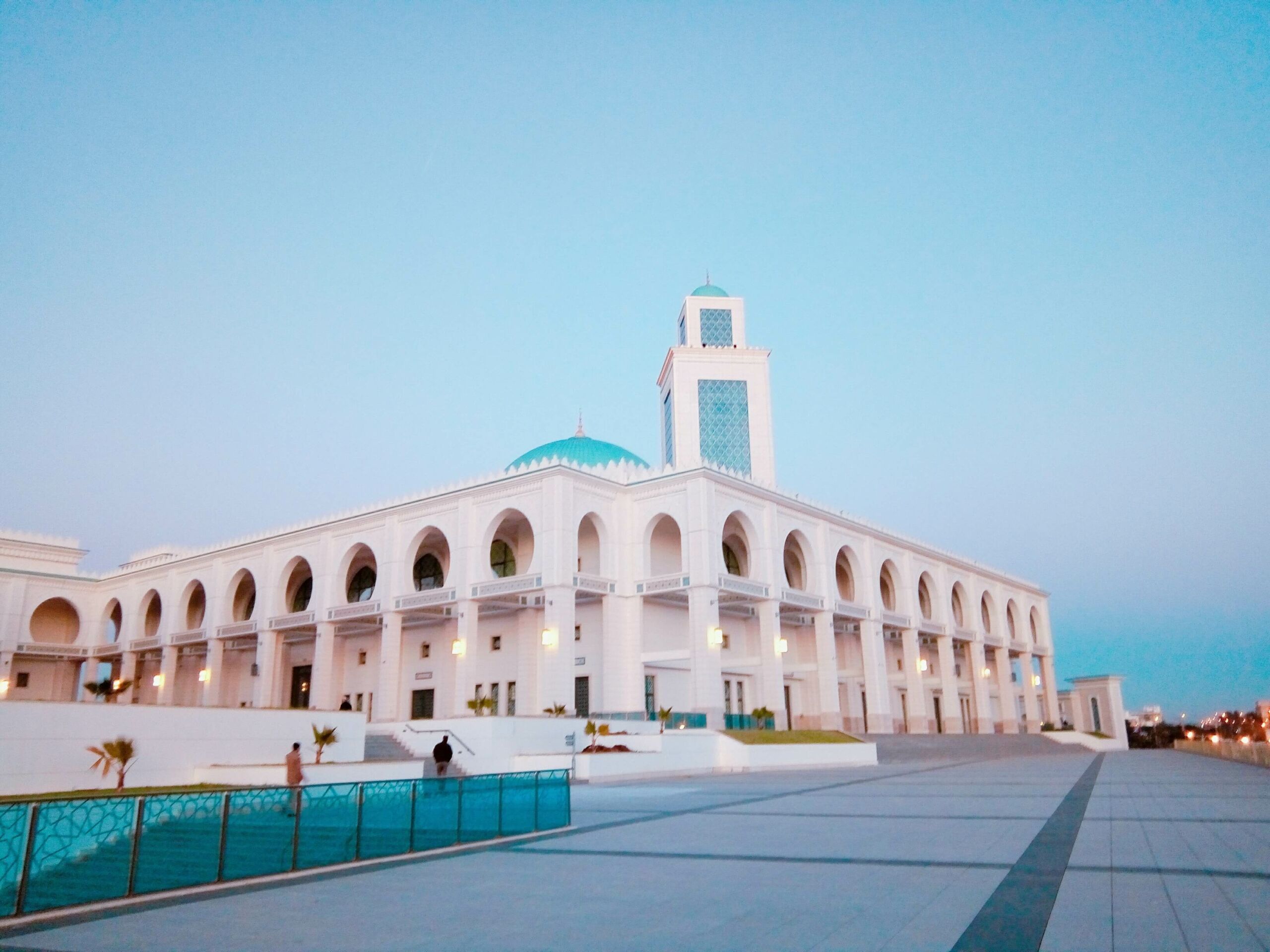
Lorem, ipsum dolor sit amet consectetur adipisicing elit. Vero labore vel temporibus quia fuga quod, voluptatem totam quidem pariatur velit earum voluptates, iusto porro, natus sapiente repudiandae? Impedit, libero delectus. Lorem ipsum dolor sit amet consectetur adipisicing elit. Deserunt reiciendis cumque nam consequatur illum veritatis doloribus aspernatur. Cupiditate perferendis, porro quod distinctio veritatis vitae fugiat odit explicabo, exercitationem alias aut. Lorem ipsum dolor sit amet, consectetur adipisicing elit.
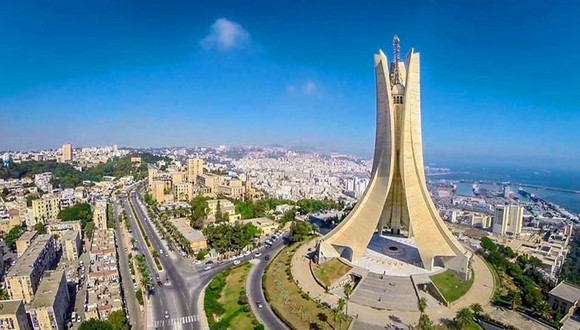
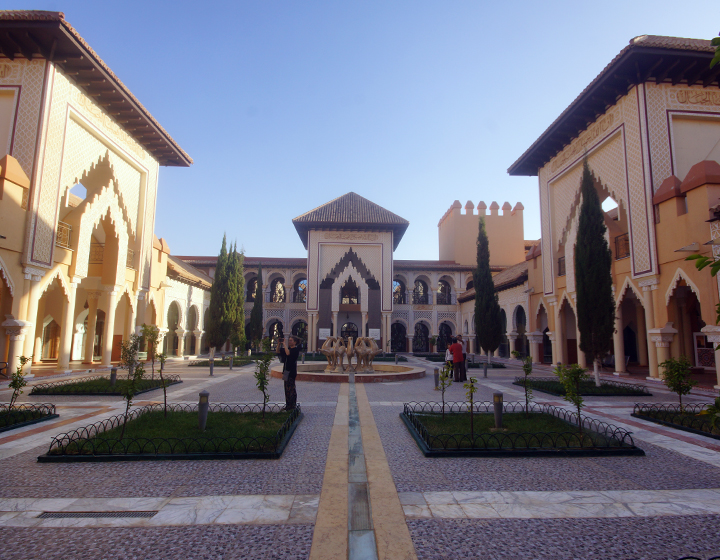
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Minima, perspiciatis labore consequuntur tenetur velit numquam odit doloribus dolor mollitia laborum facere optio aperiam magnam, unde vel dolores accusamus. Nihil, harum?. Lorem ipsum dolor sit amet, consectetur adipisicing elit. Minima, perspiciatis labore consequuntur tenetur velit numquam odit doloribus dolor mollitia laborum facere optio aperiam magnam, unde vel dolores accusamus. Nihil, harum? Lorem ipsum dolor sit amet, consectetur adipisicing elit.
FlexBox
Flexbox—formally Flexible Box Layout—is a new method for laying out
elements on the page It introduces 12 new properties to CSS,
including some shorthand properties.
Flexbox begins with the familiar display property. Applying display: flex to an element
turns it into a flex container, and its direct children turn into
flex items.
By default, flex items align side by side, left to
right, all in one row. The flex container fills the available width
like a block element, but the flex items may not necessarily fill
the width of their flex container.
The flex items are all the same
height, determined naturally by their contents. You can also use
display: inline-flex.
Flex direction
This specifies the direction of the main axis. The cross axis will be perpendicular to the main axis.
<-----------Main axis----------->
<------Cross axis------>
HTML
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
display: flex;
flex-direction: row;
}
Result
flex-direction: row (default)
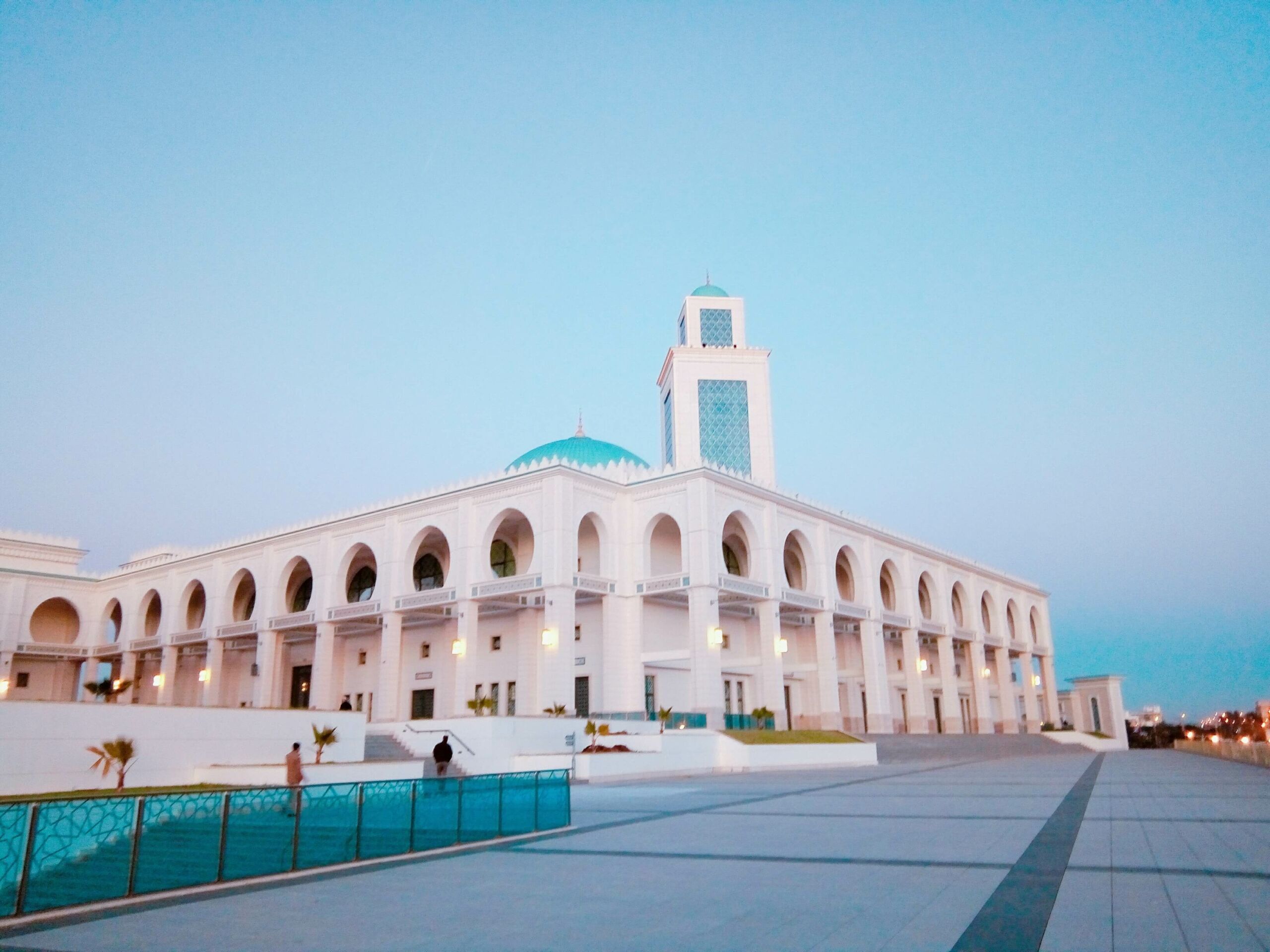
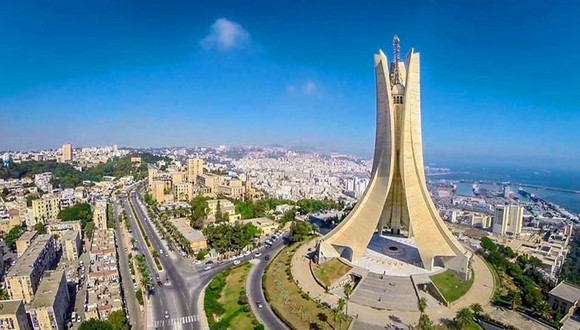
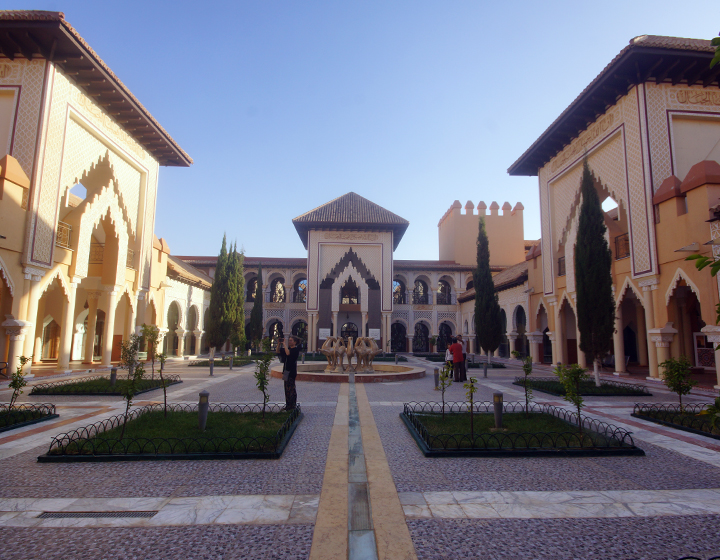
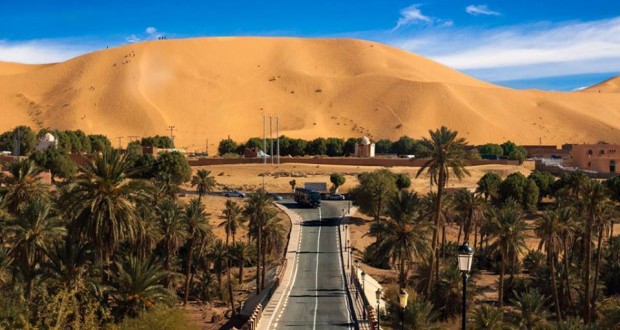
flex-direction: row-reverse
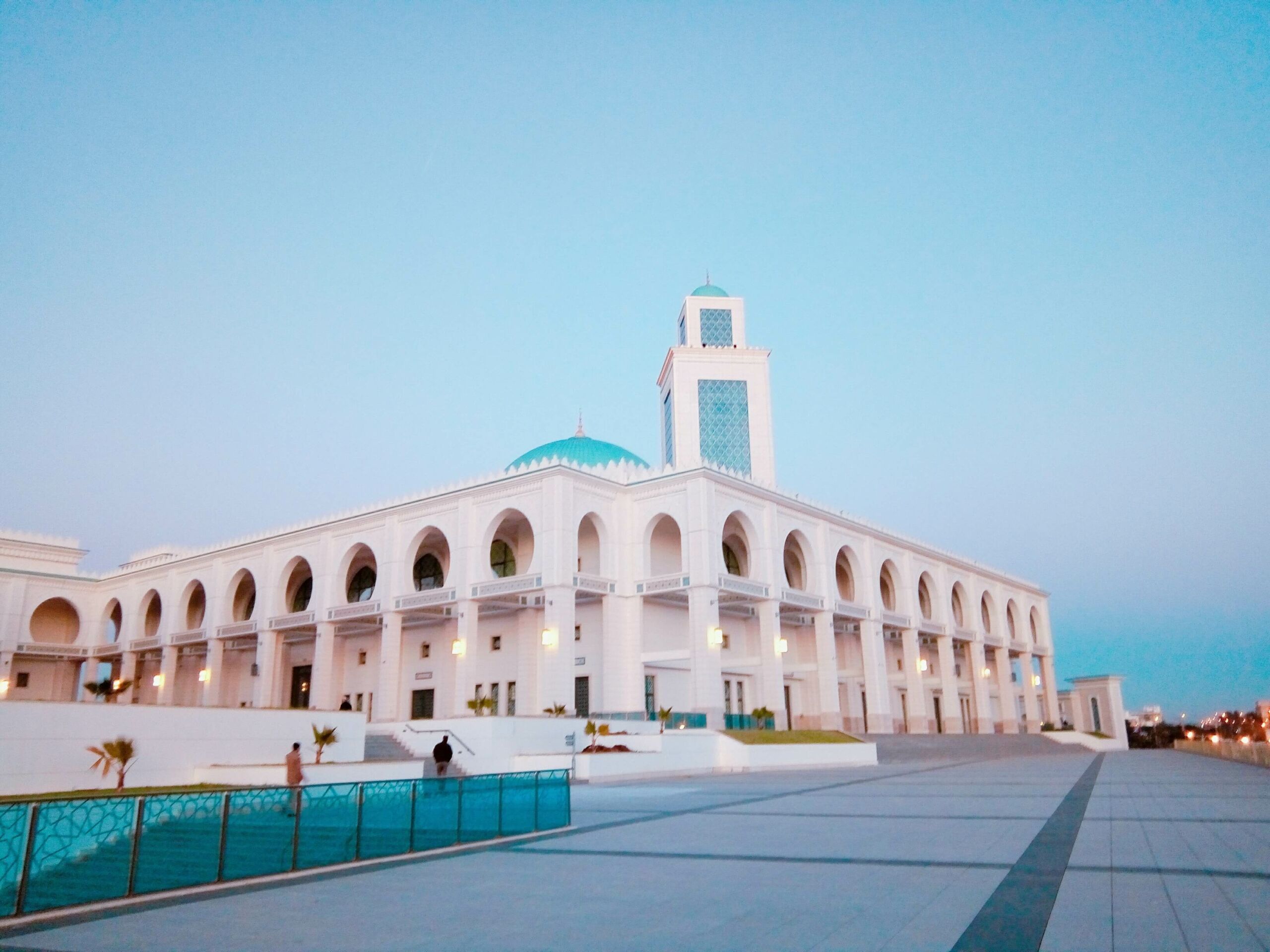
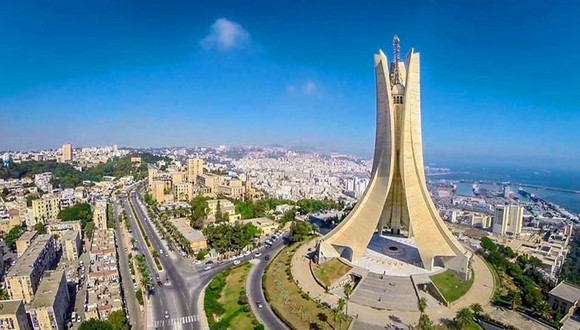
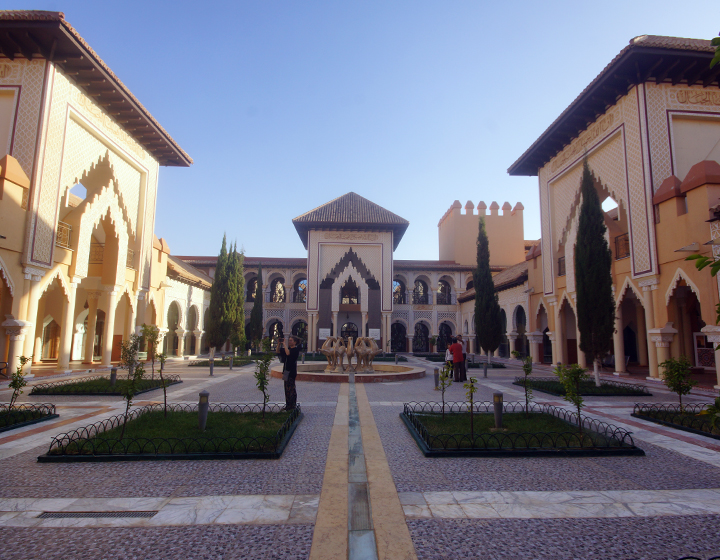
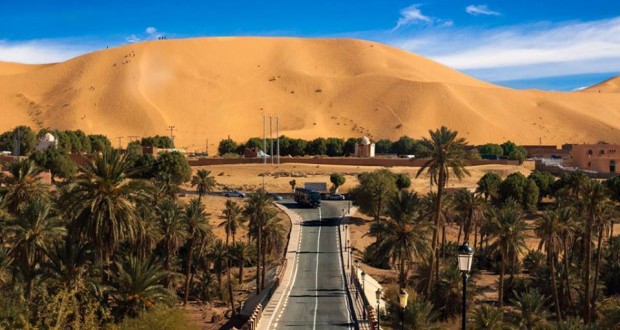
HTML
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
display: flex;
flex-direction: column;
}
Result
flex-direction: column
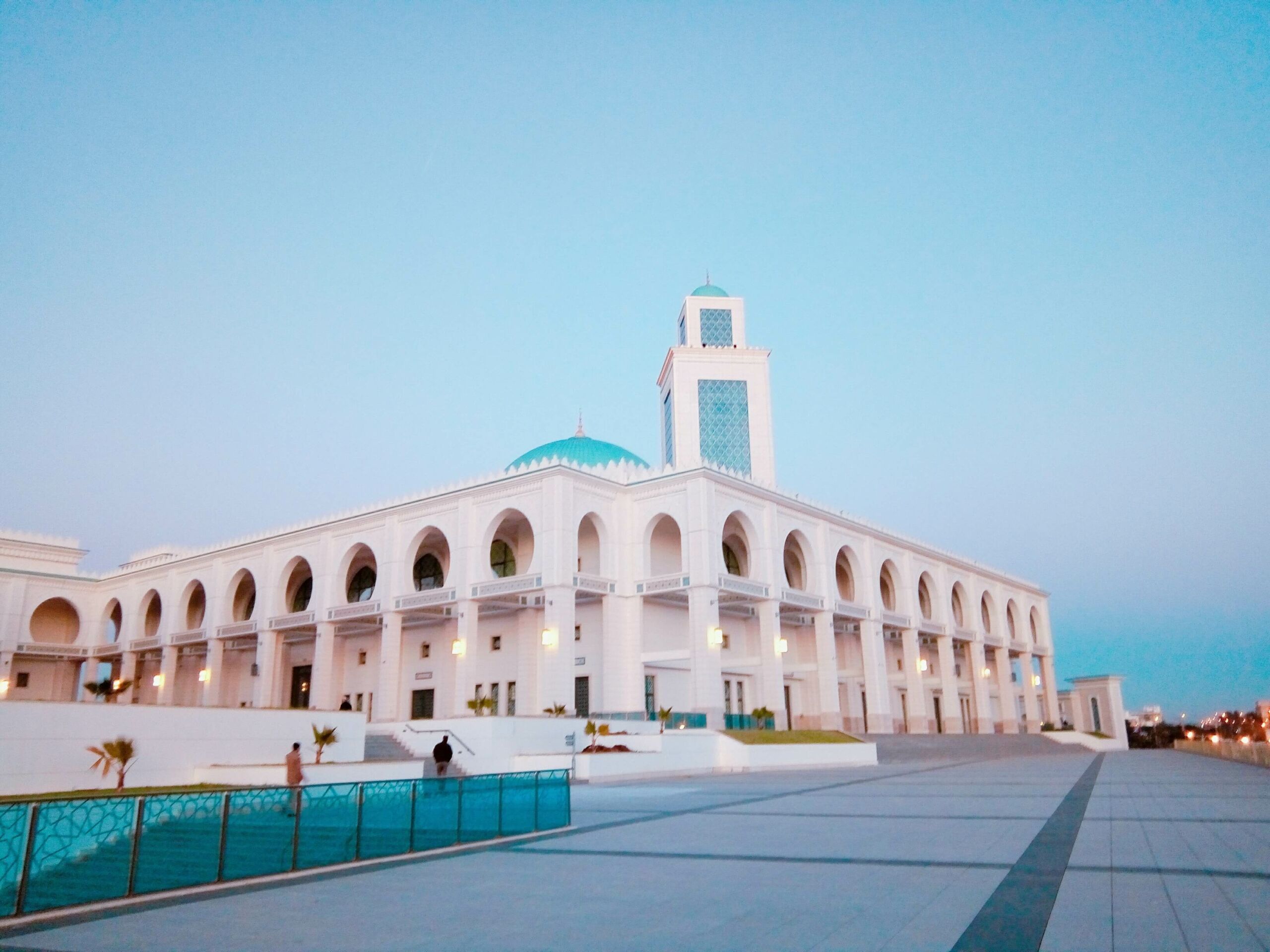
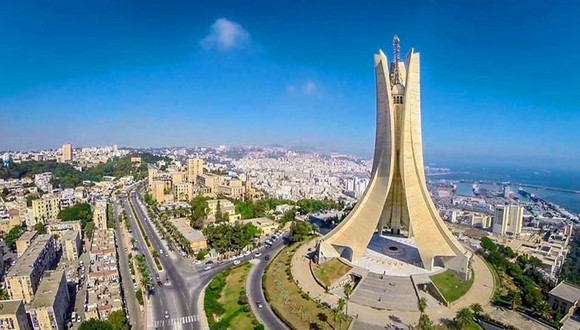
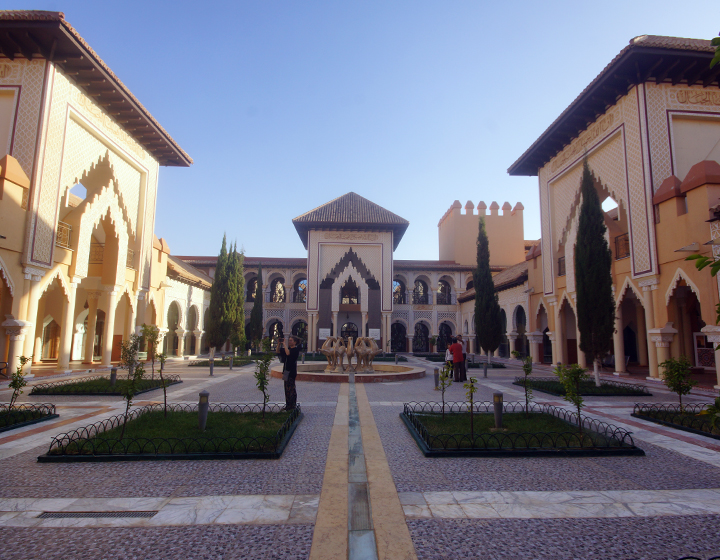
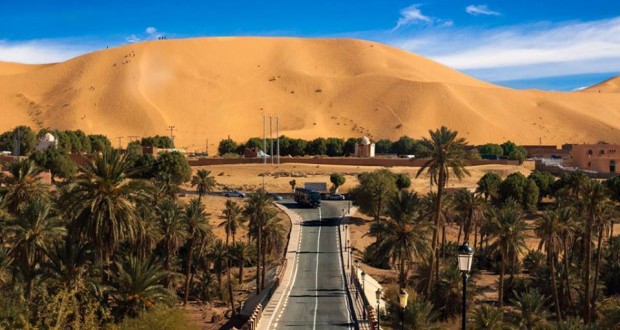
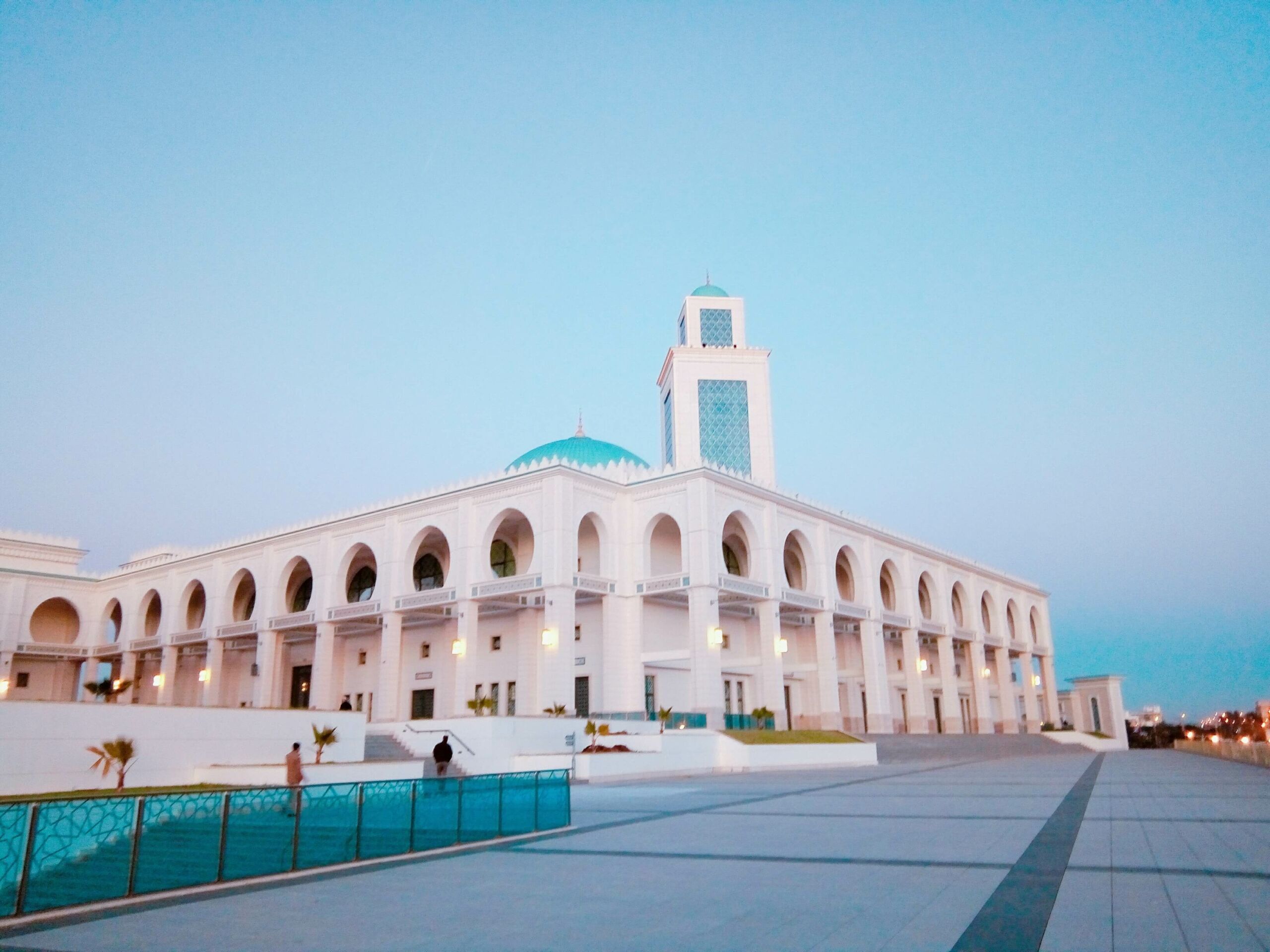
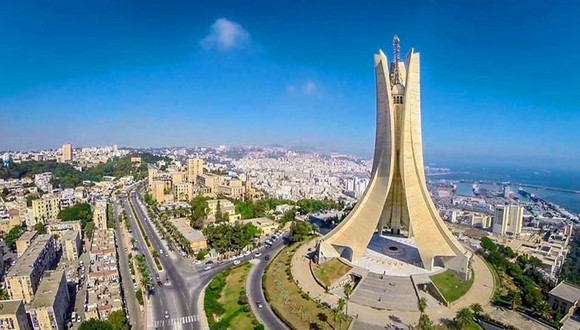
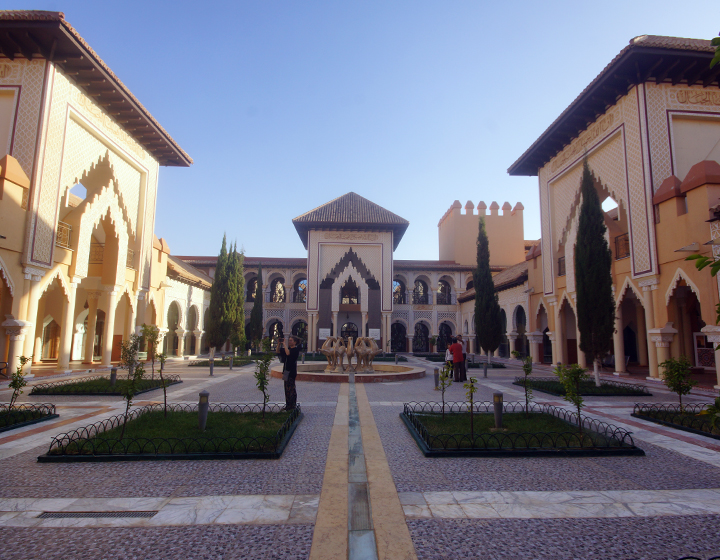
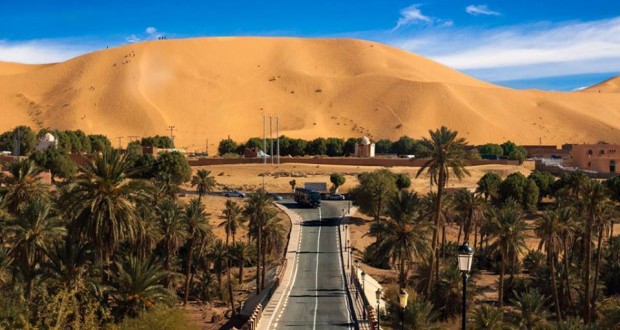
flex-direction: column-reverse
Flex wrap
This specifies whether flex items will wrap on to a new row inside
the flex container (or on to a new column if flex-direction is
column or column-reverse).
Also flex-flow is a shorthand for flex-direction flex-wrap
HTML
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
display: flex;
flex-wrap: nowrap;
}
Result
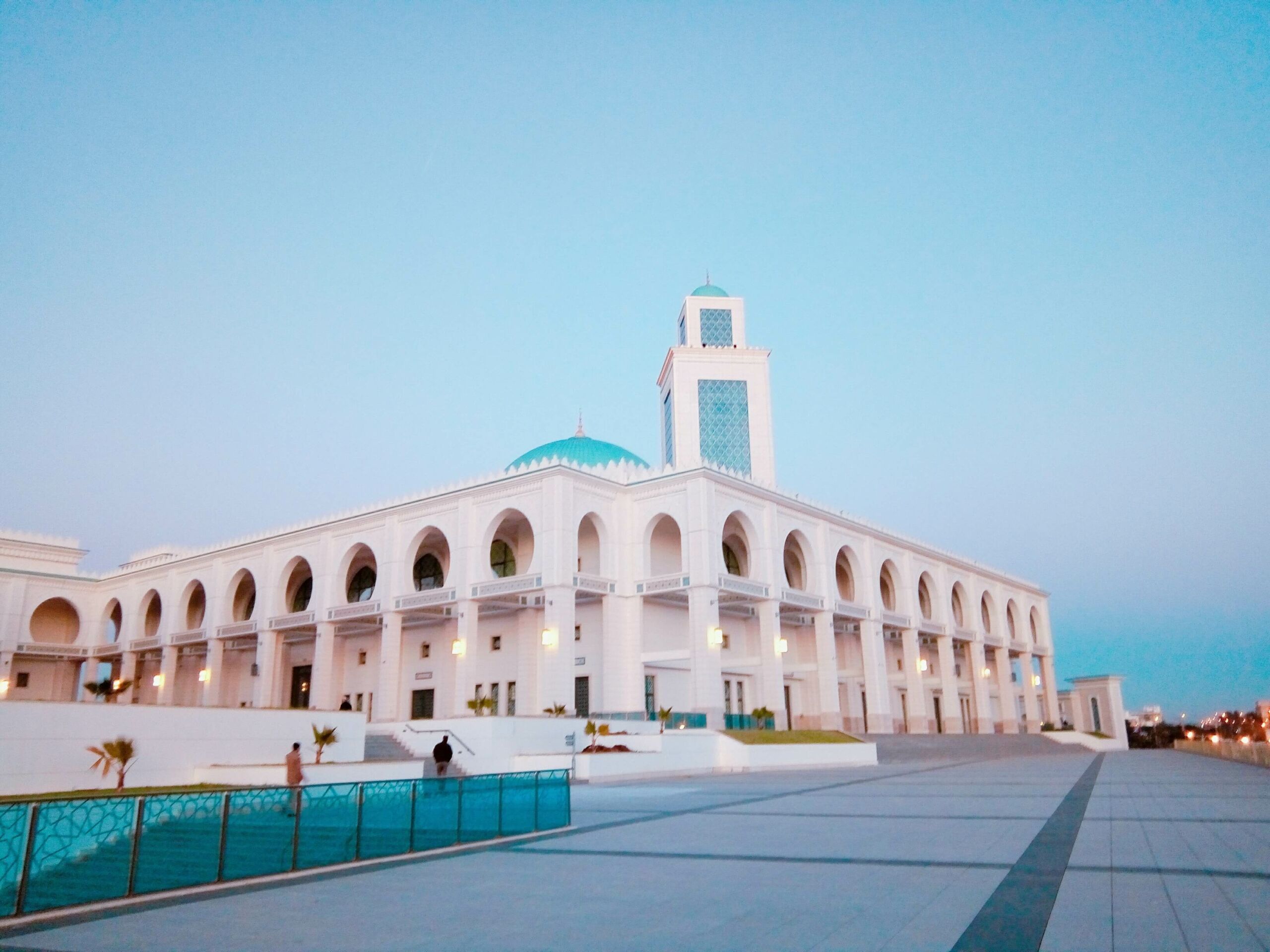
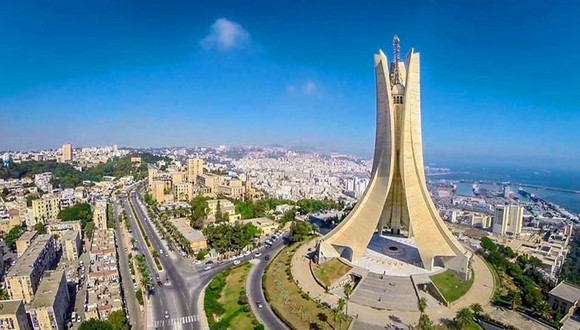
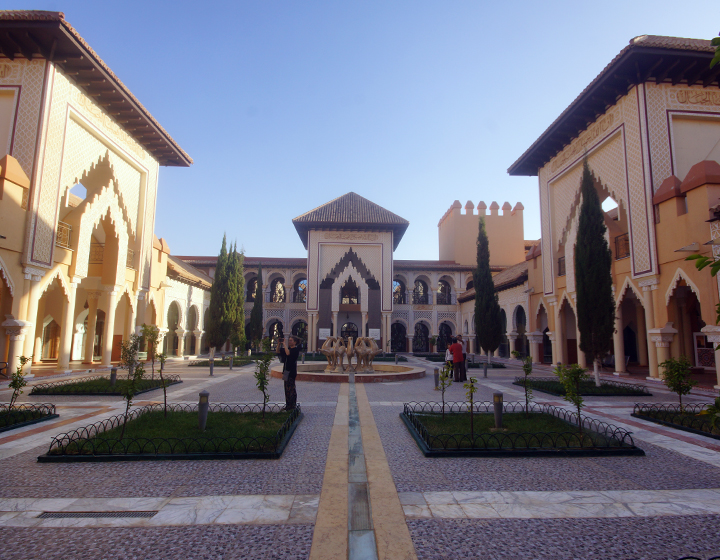
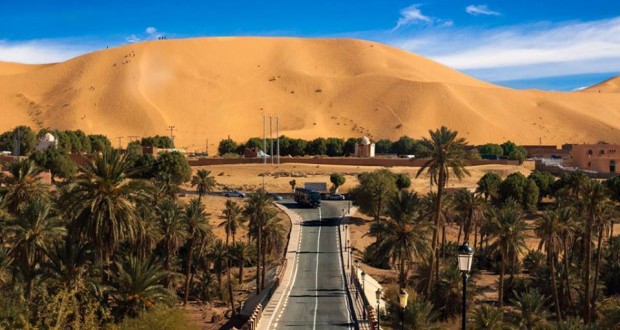
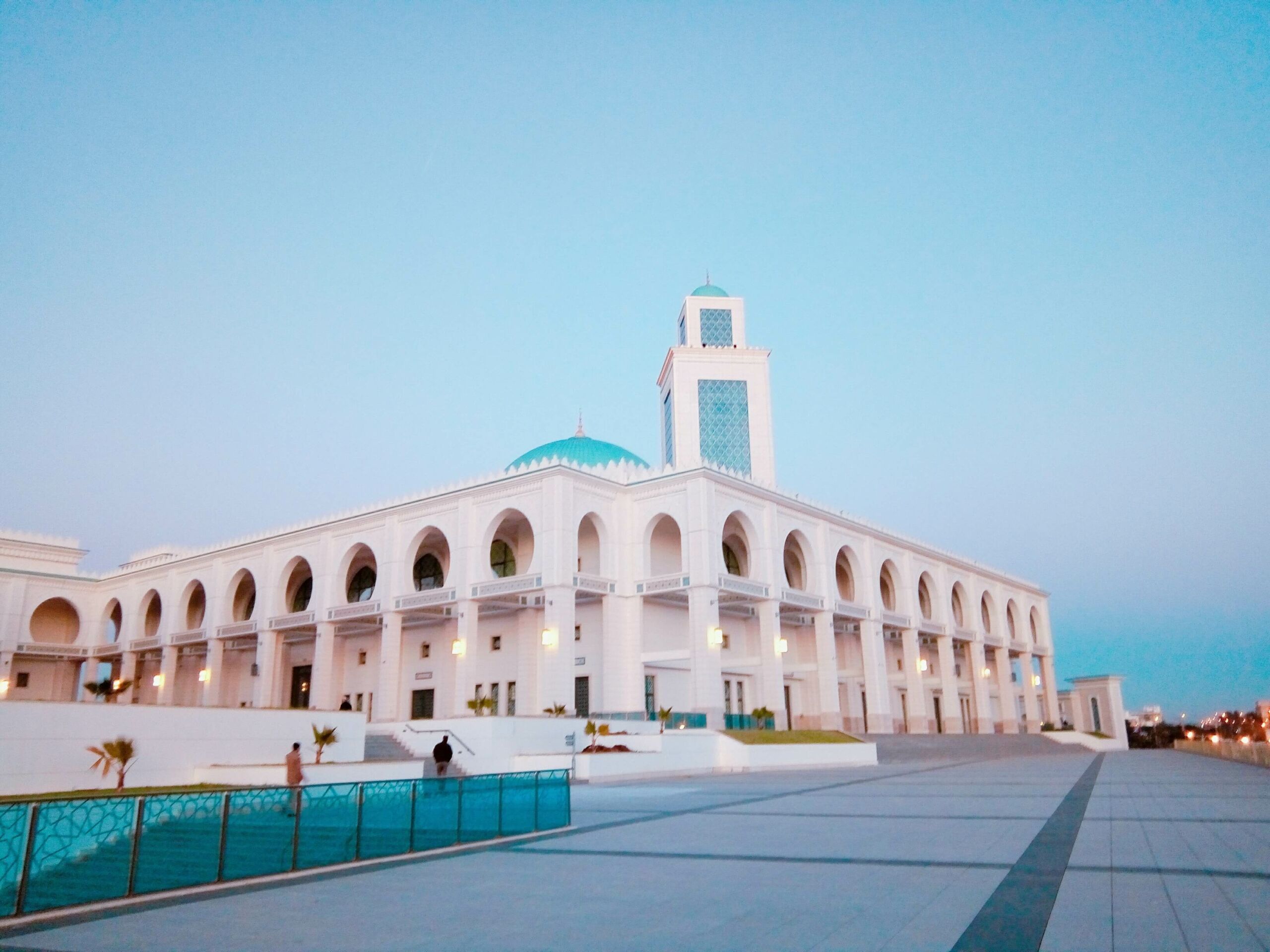
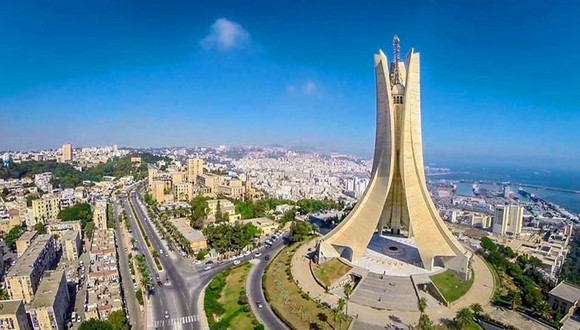
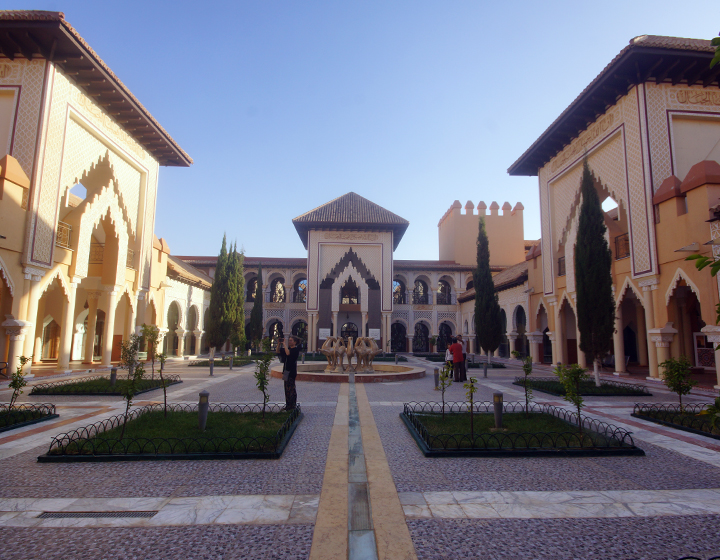
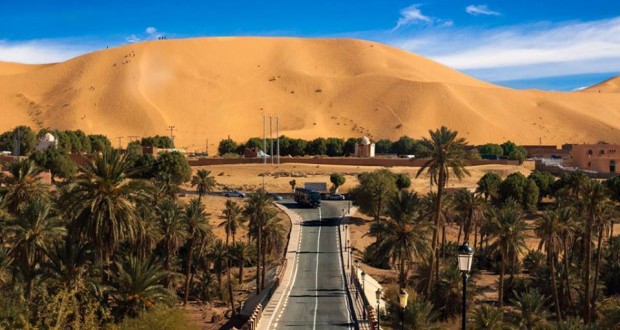
flex-wrap: nowrap
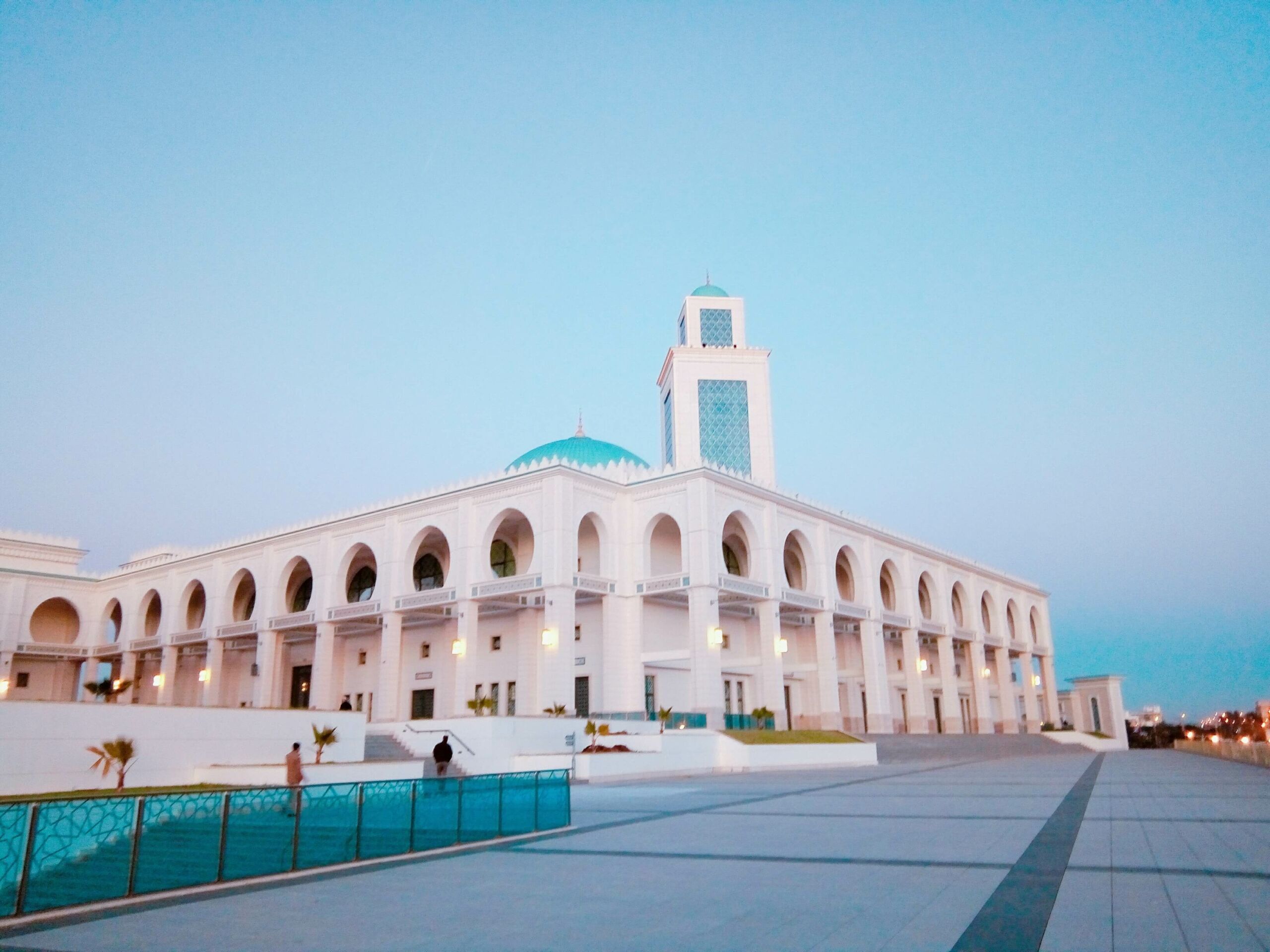
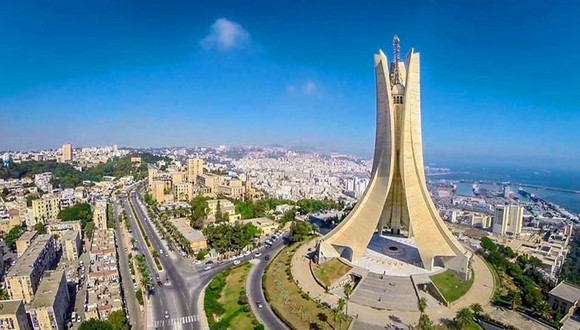
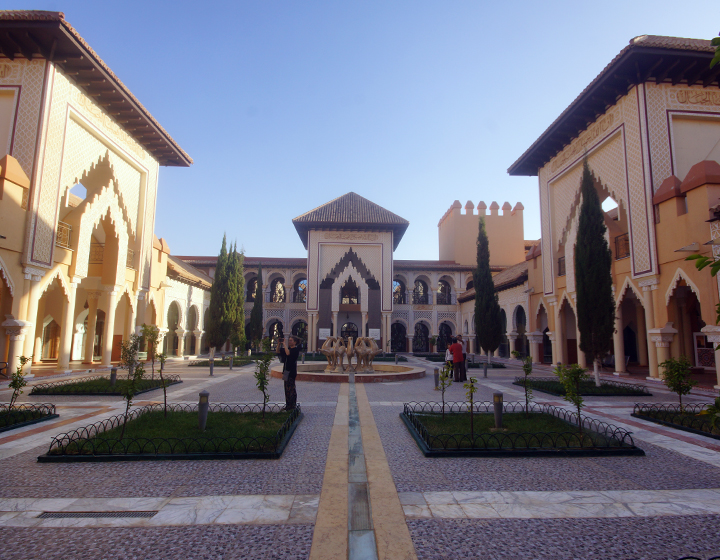
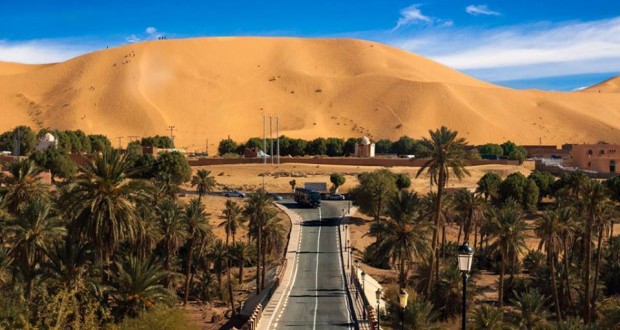
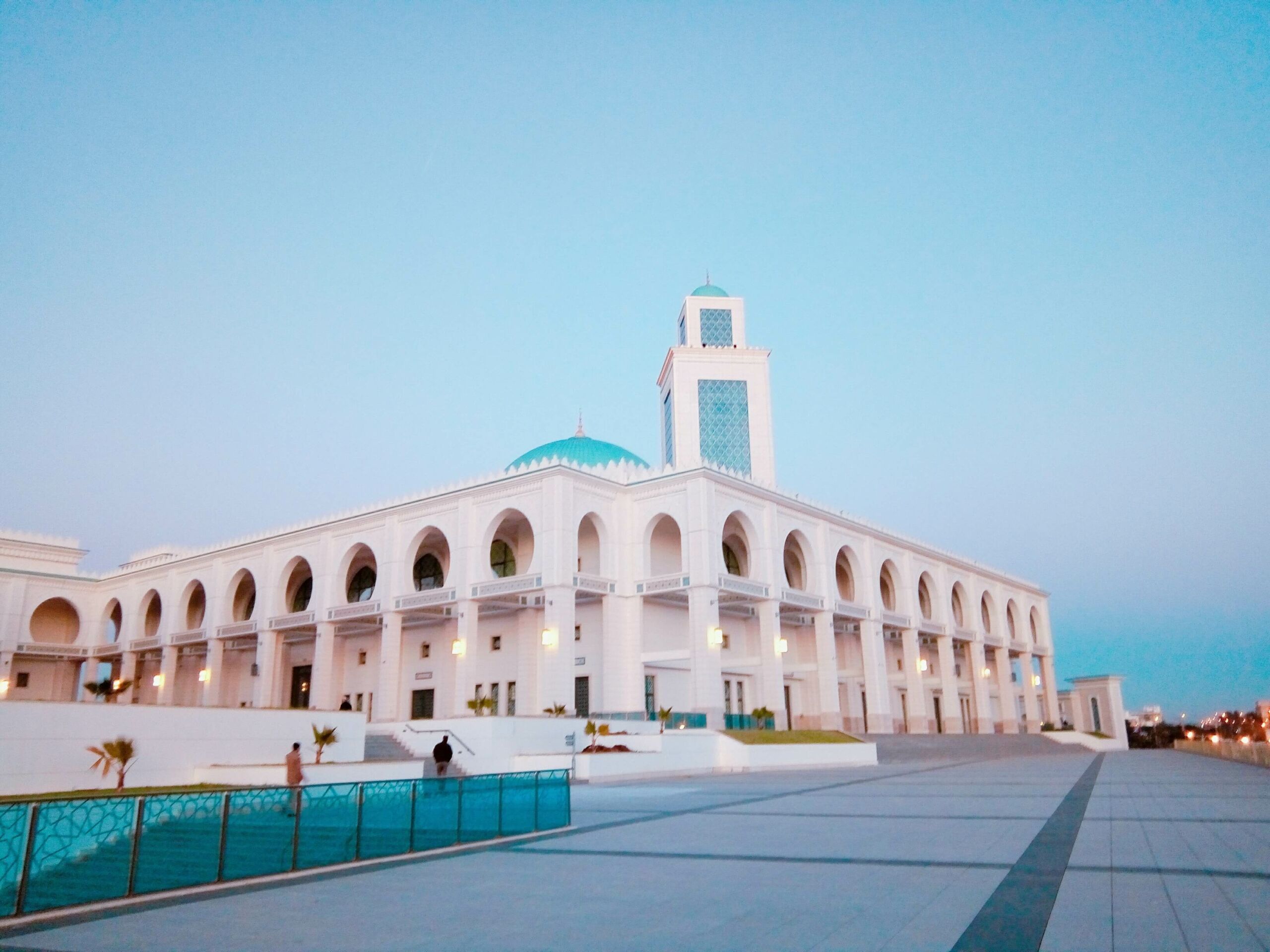
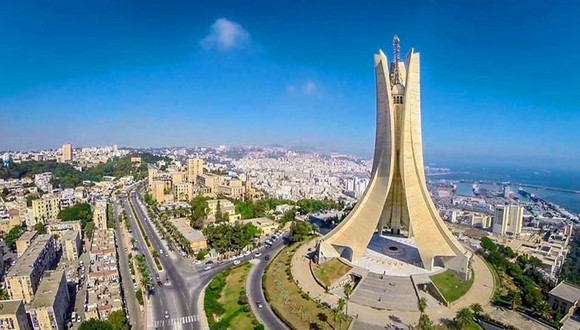
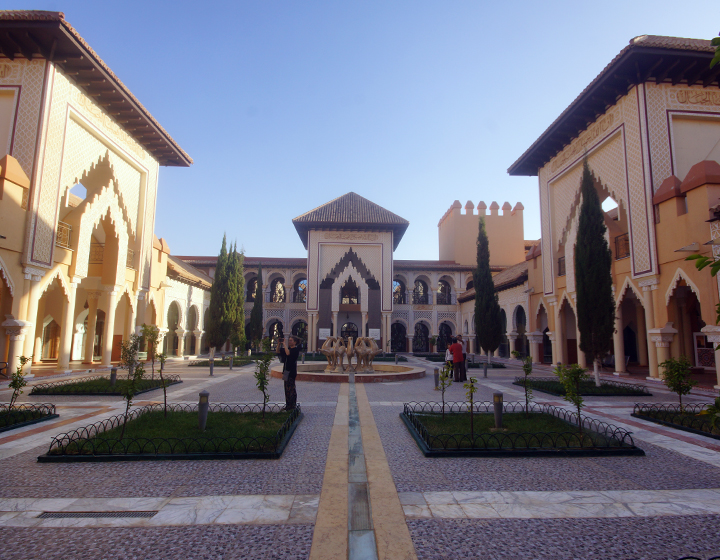
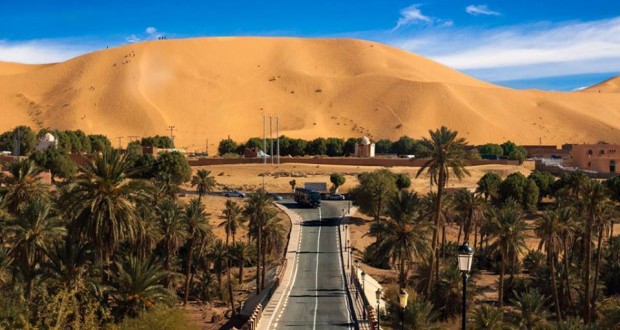
flex-wrap: wrap
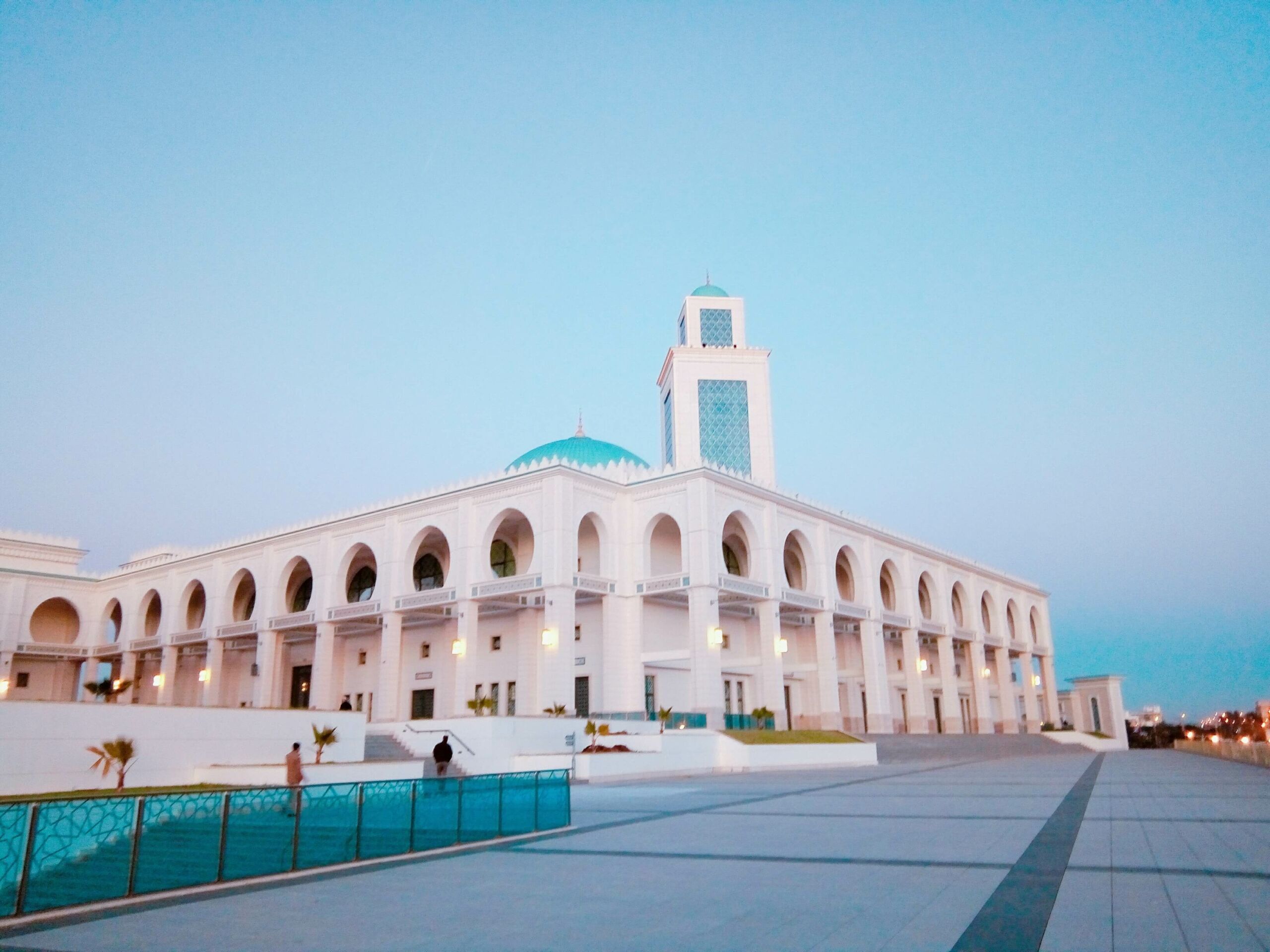
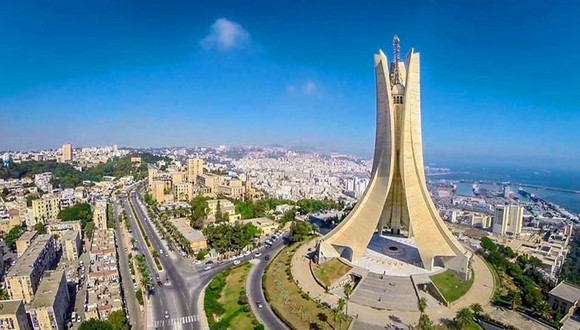
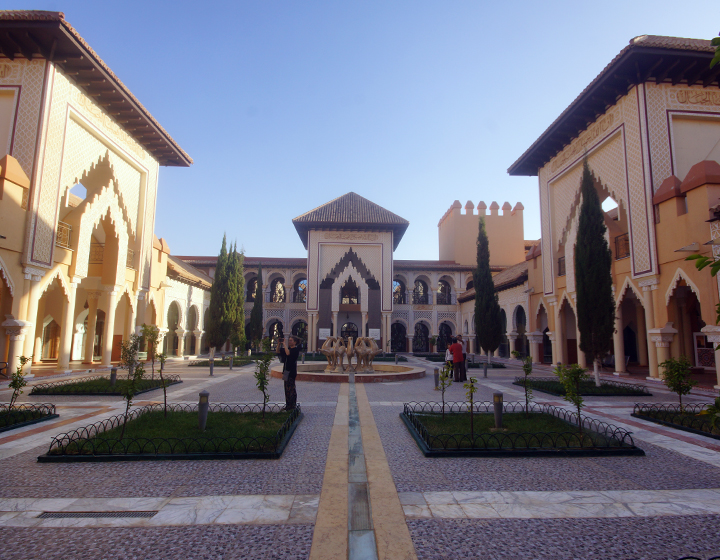
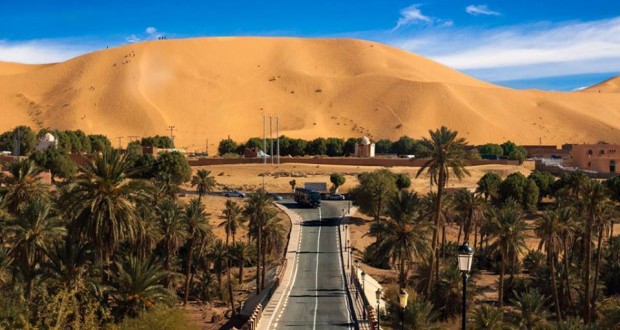
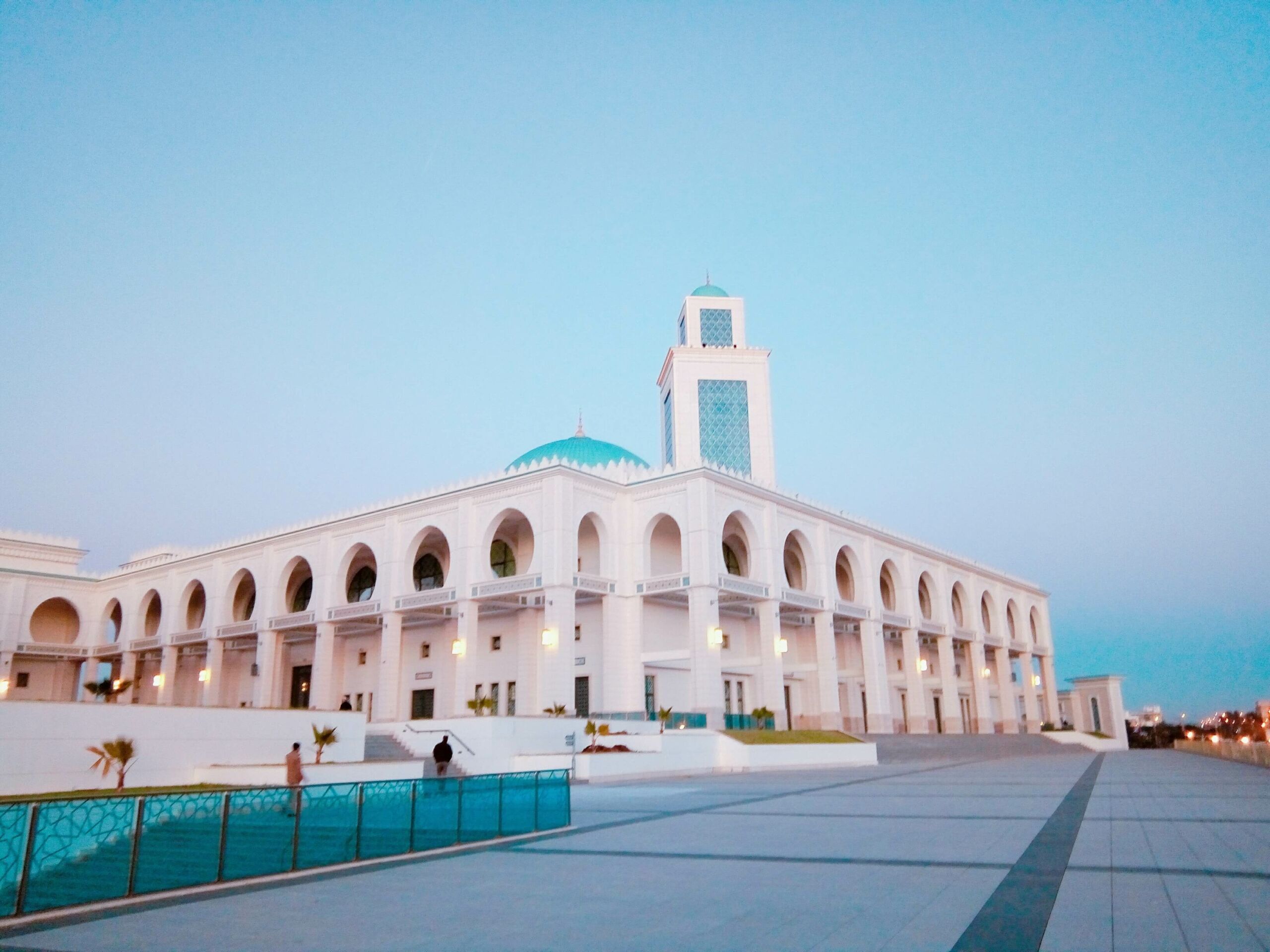
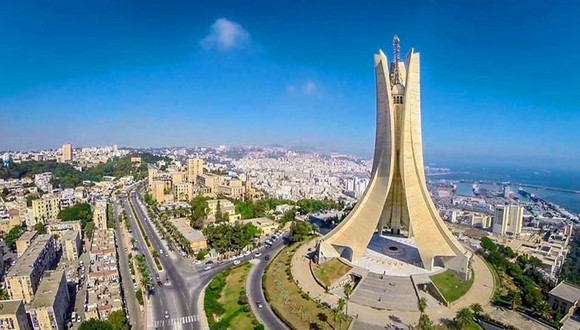
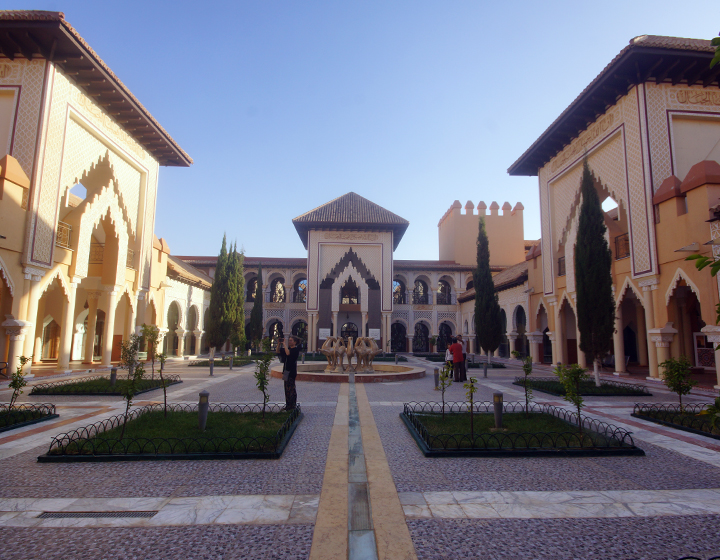
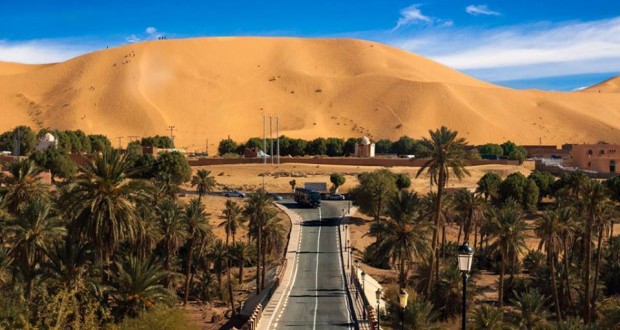
flex-wrap: wrap-reverse
justify-content
Controls how items are positioned along the main axis.
HTML
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
display: flex;
justify-content: flex-start;
}
Result
justify-content: flex-start
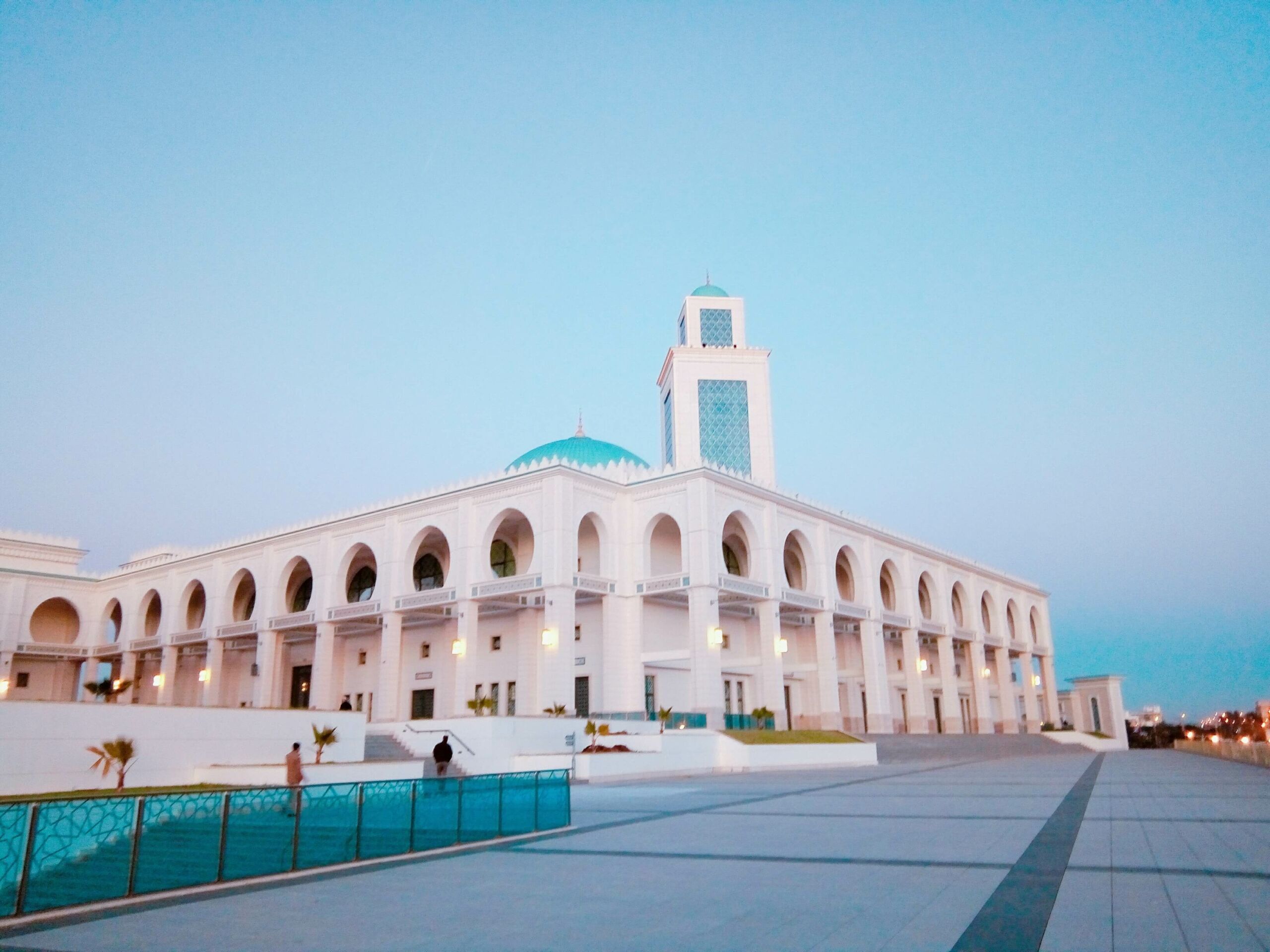
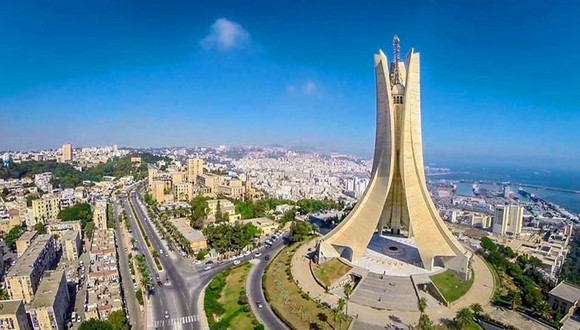
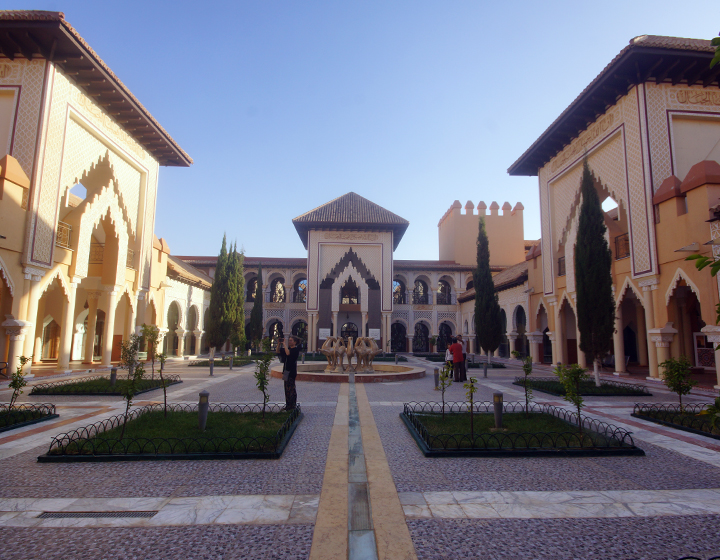
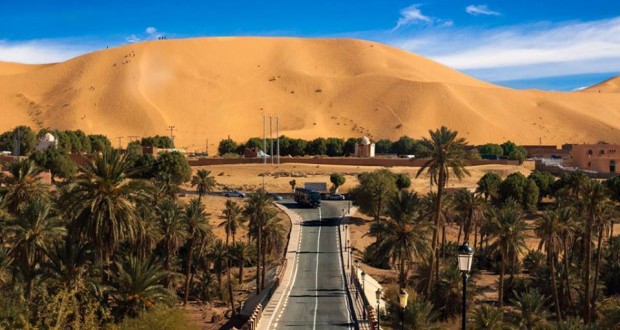
justify-content: flex-end
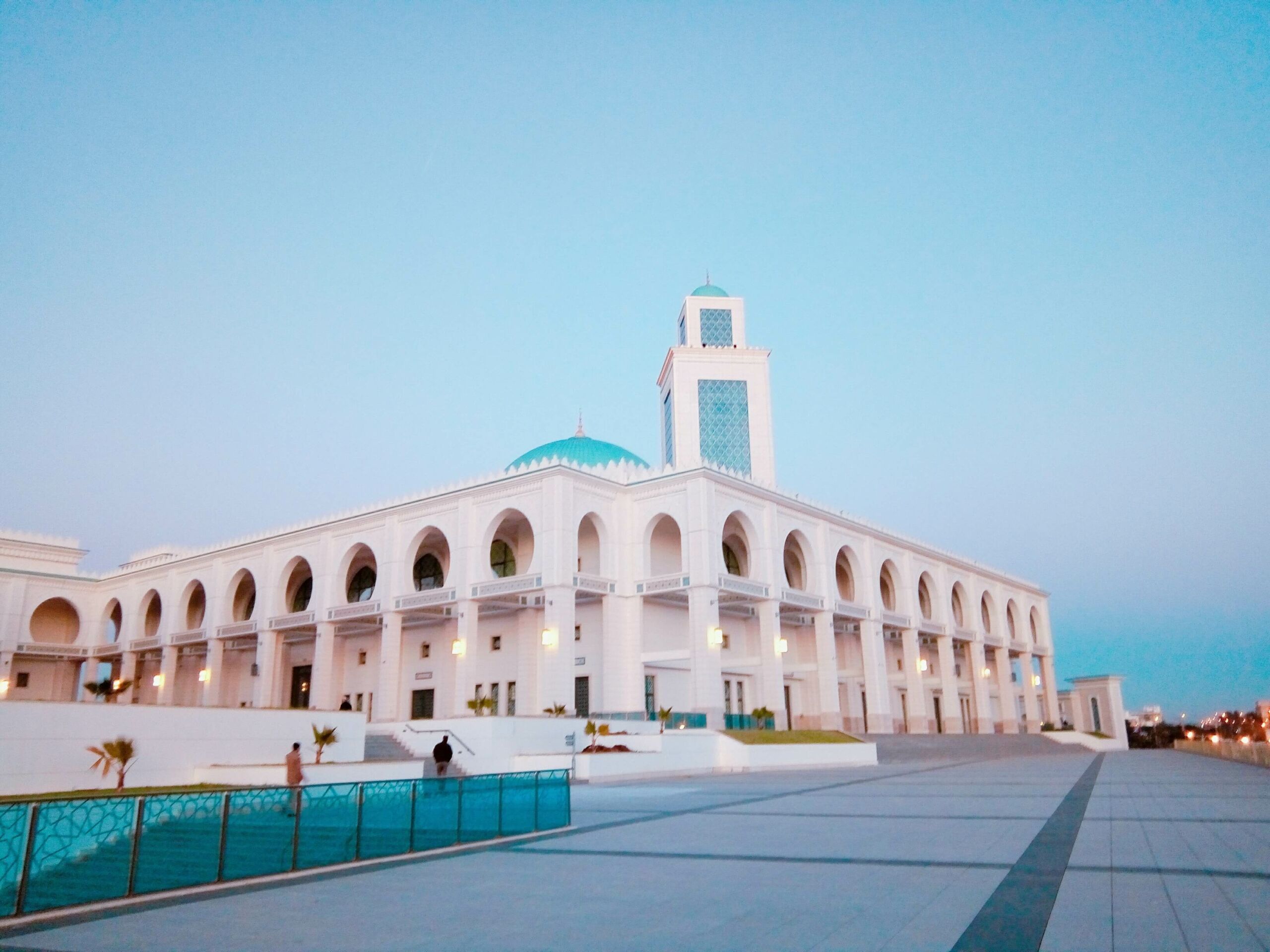
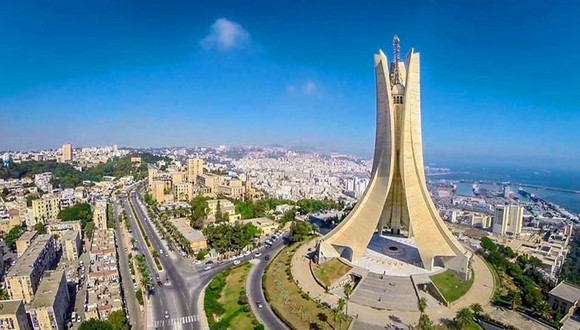
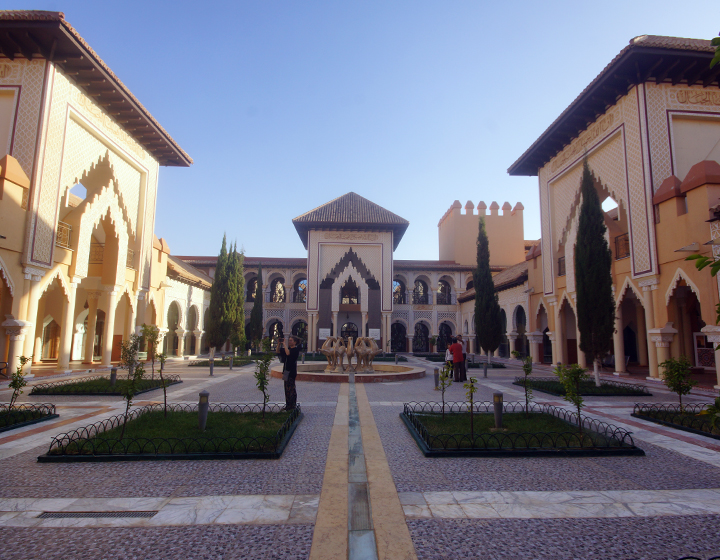
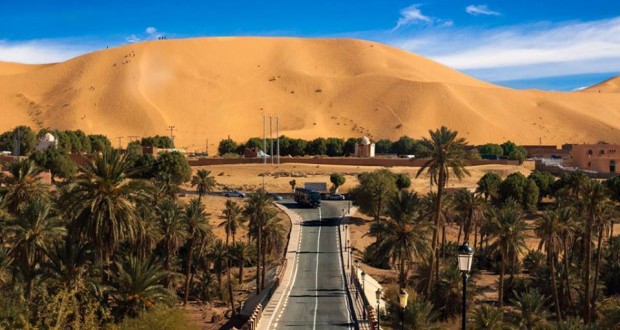
justify-content: center
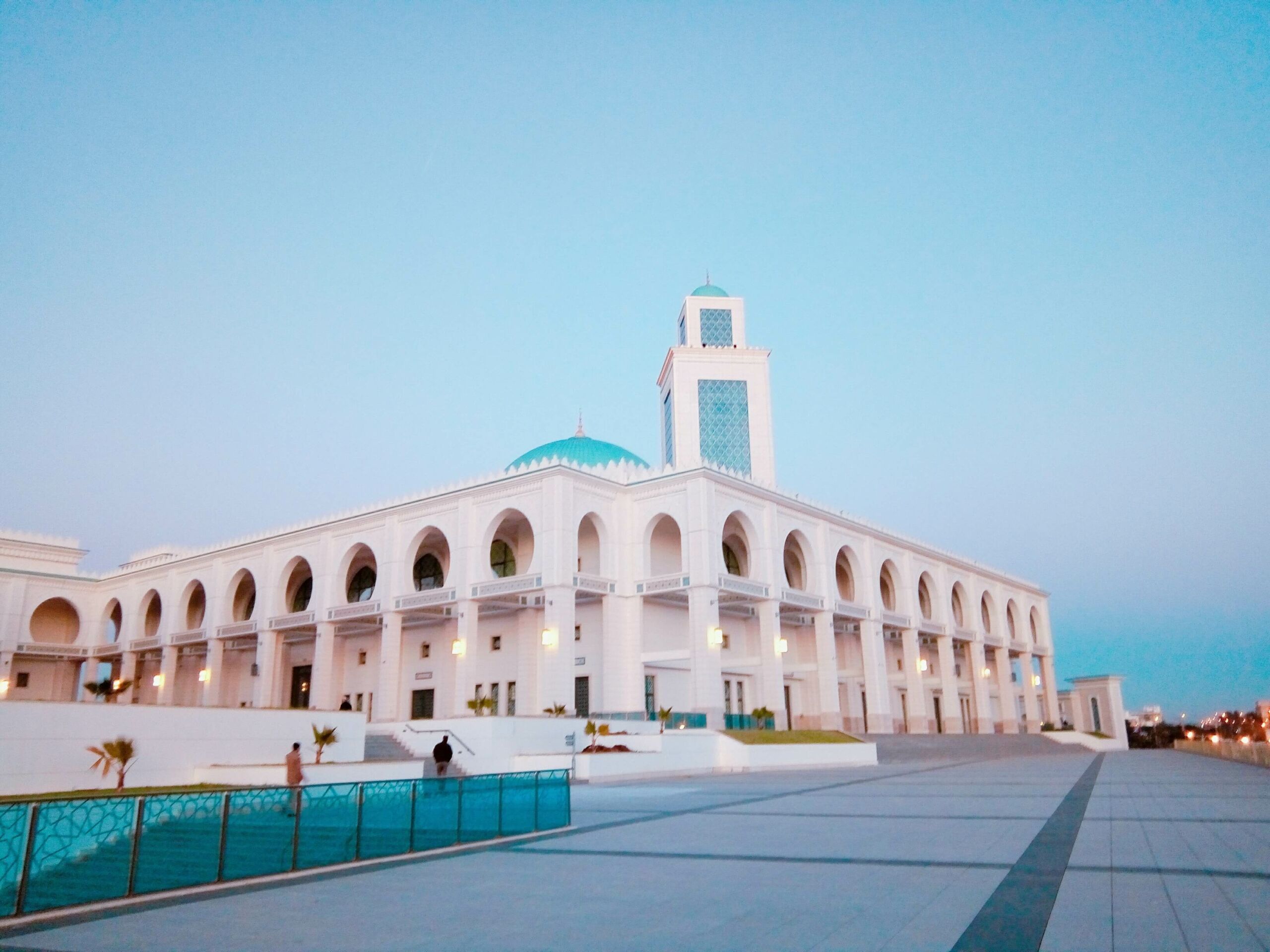
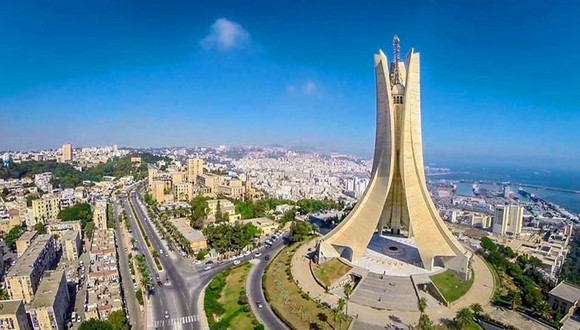
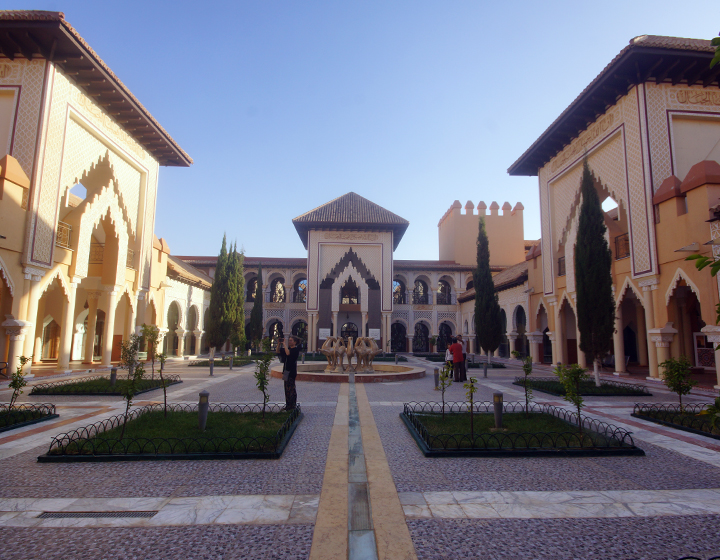
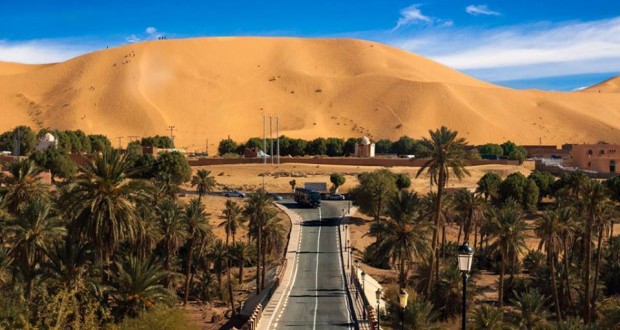
justify-content: space-between
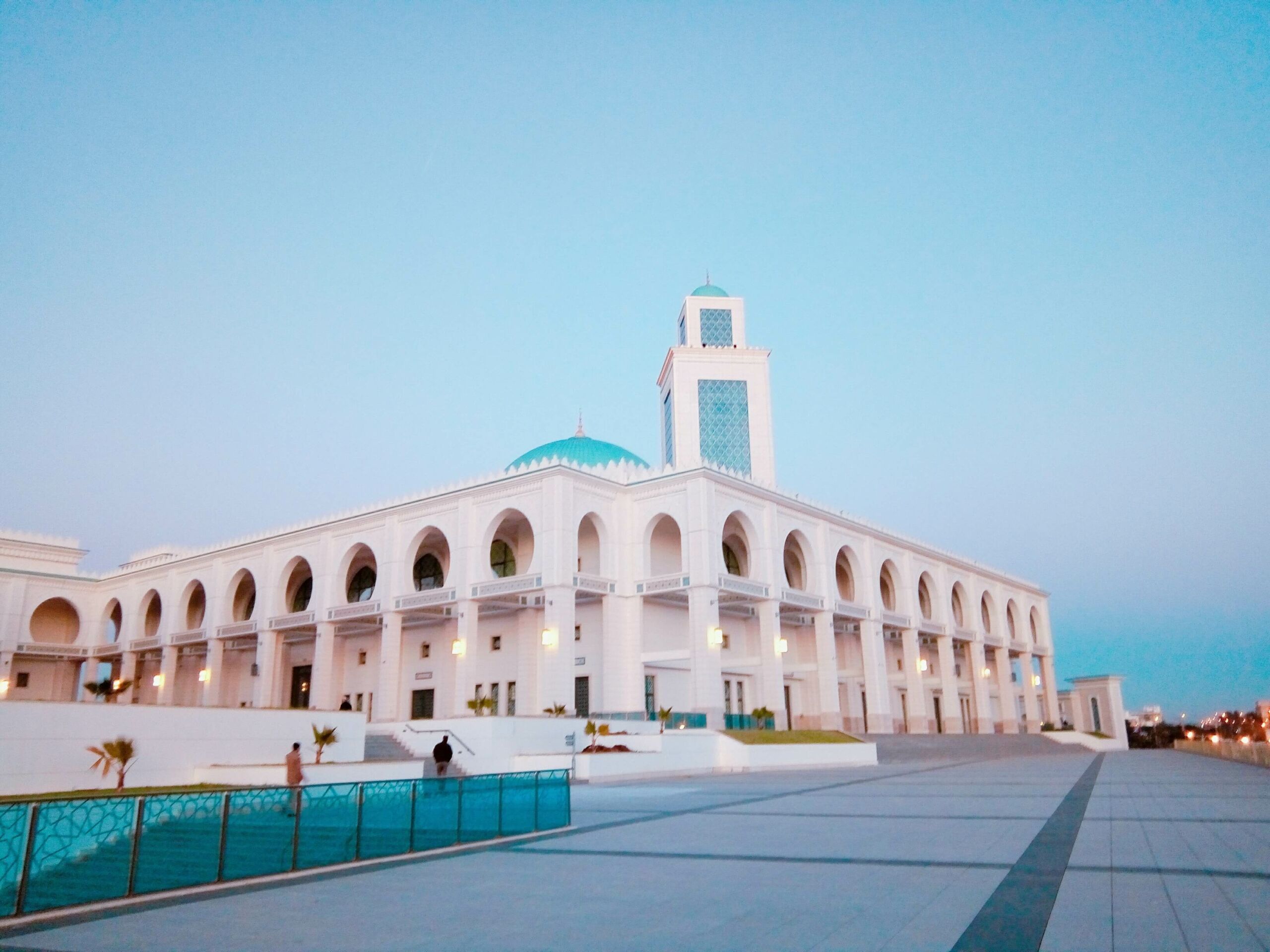
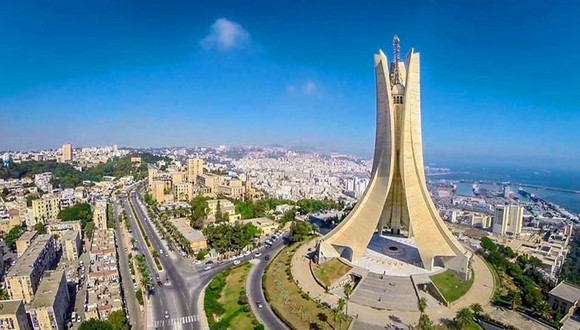
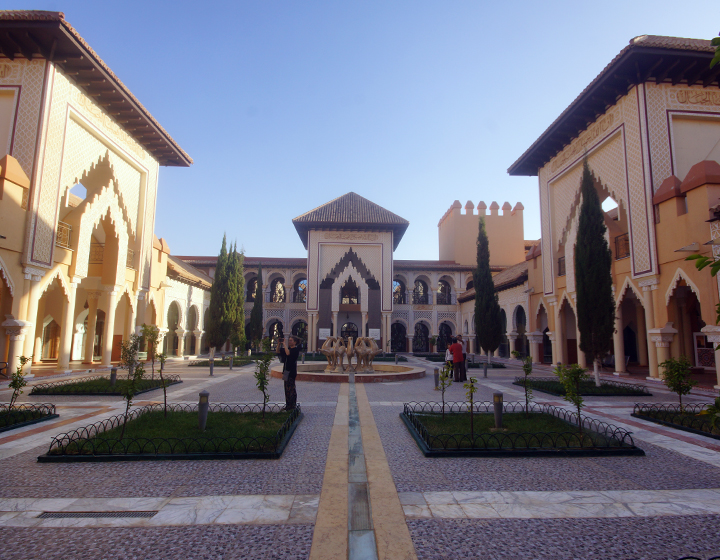
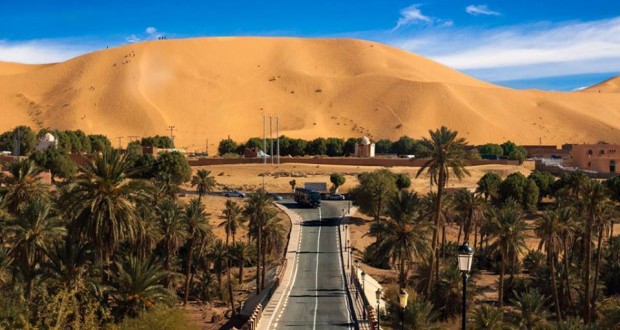
justify-content: space-around
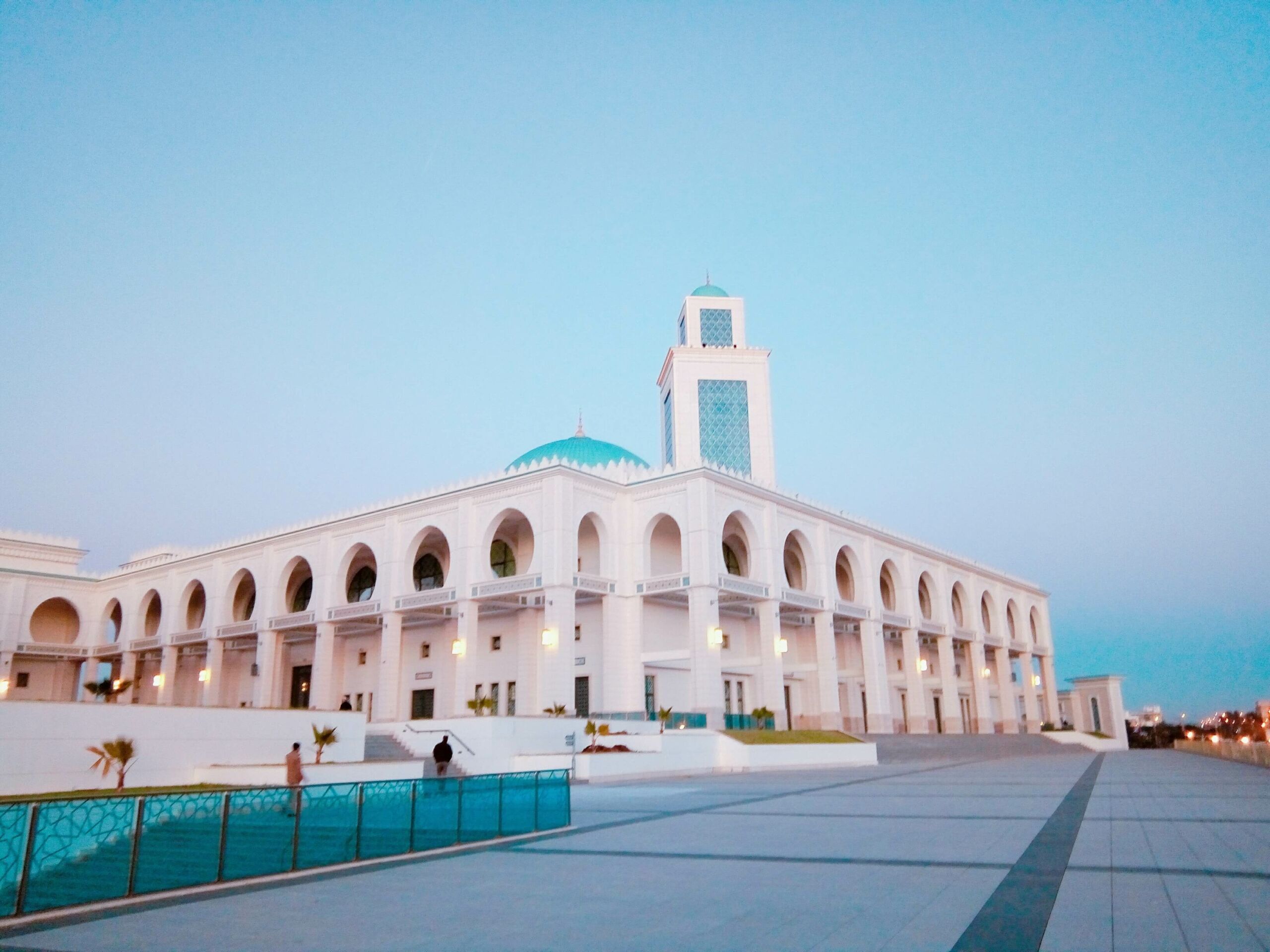
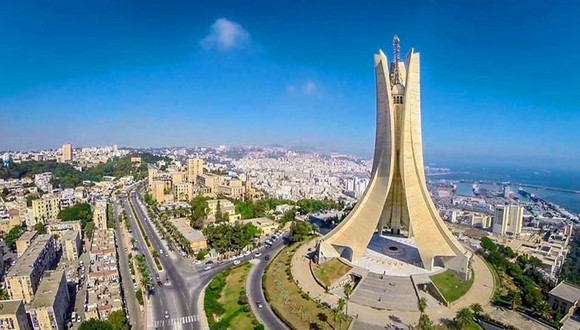
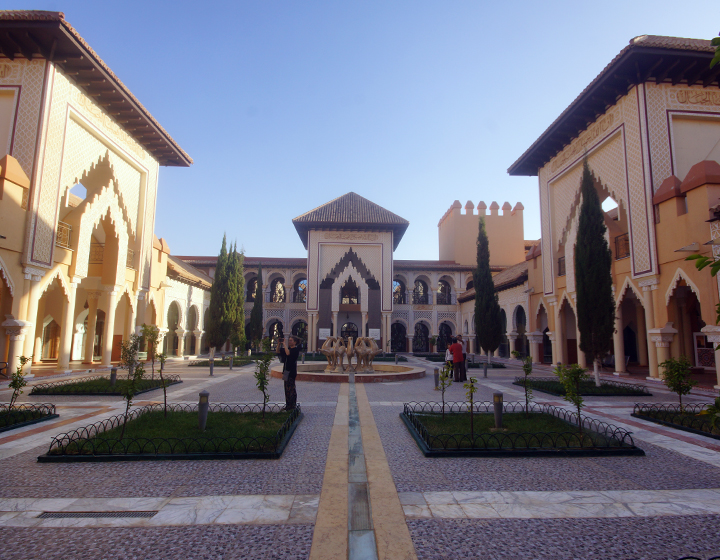
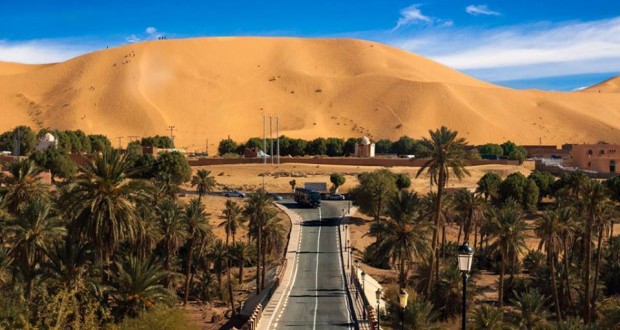
justify-content: space-evenly
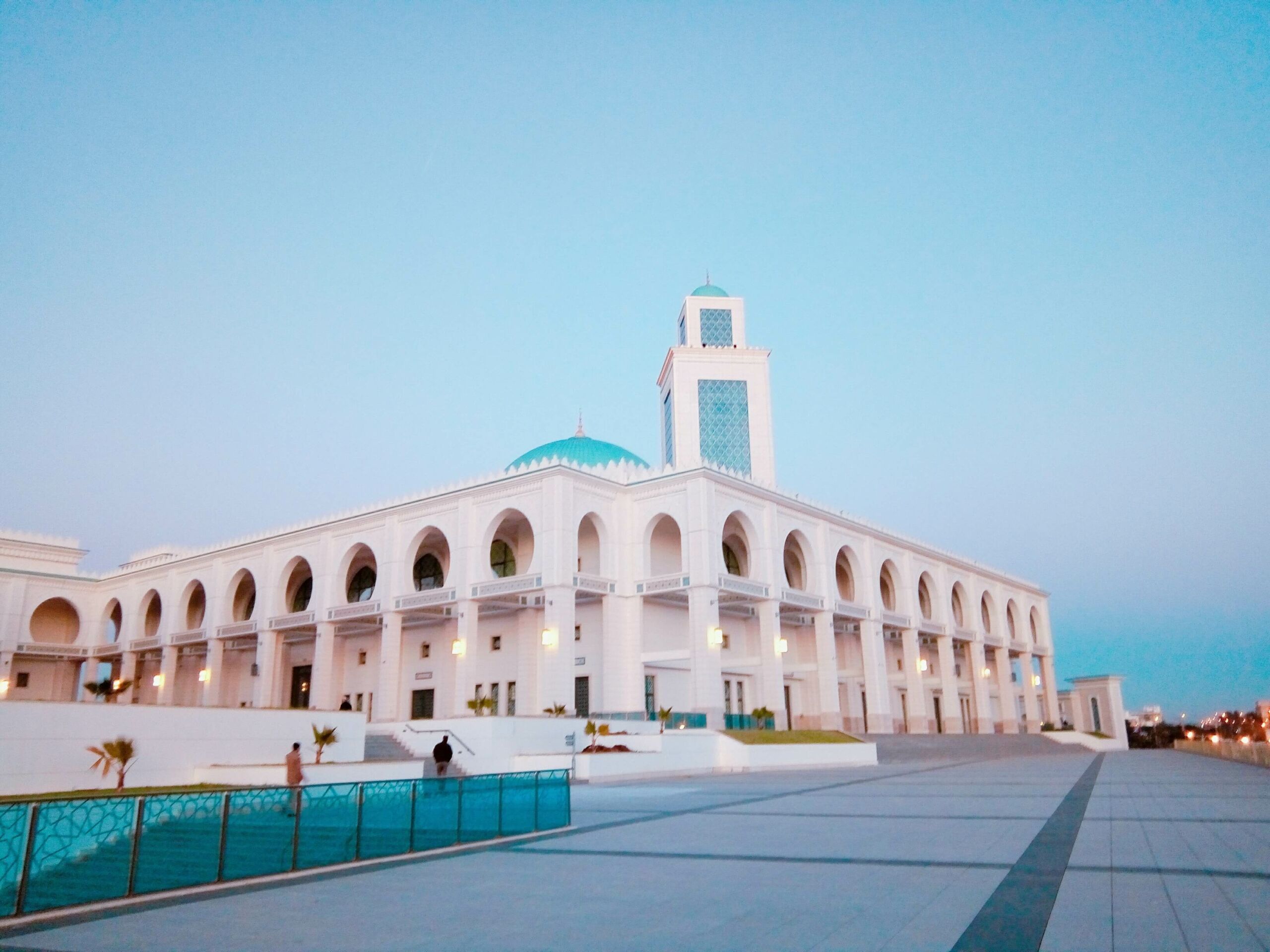
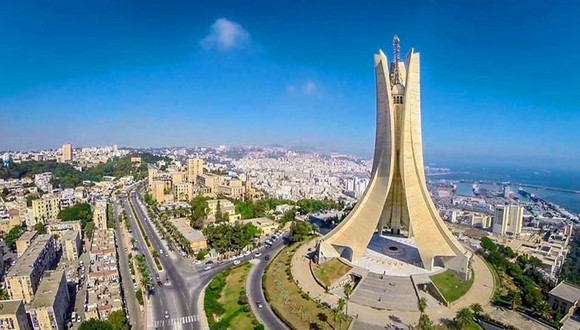
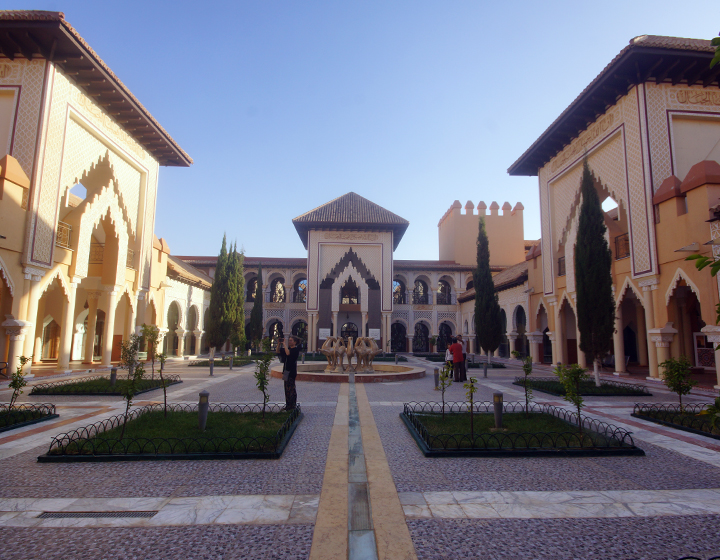
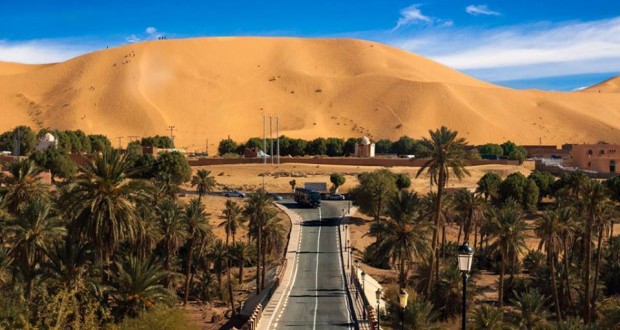
align-items
Controls how items are positioned along the cross axis.
HTML
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
display: flex;
align-items: flex-start;
}
Result
align-items: flex-start
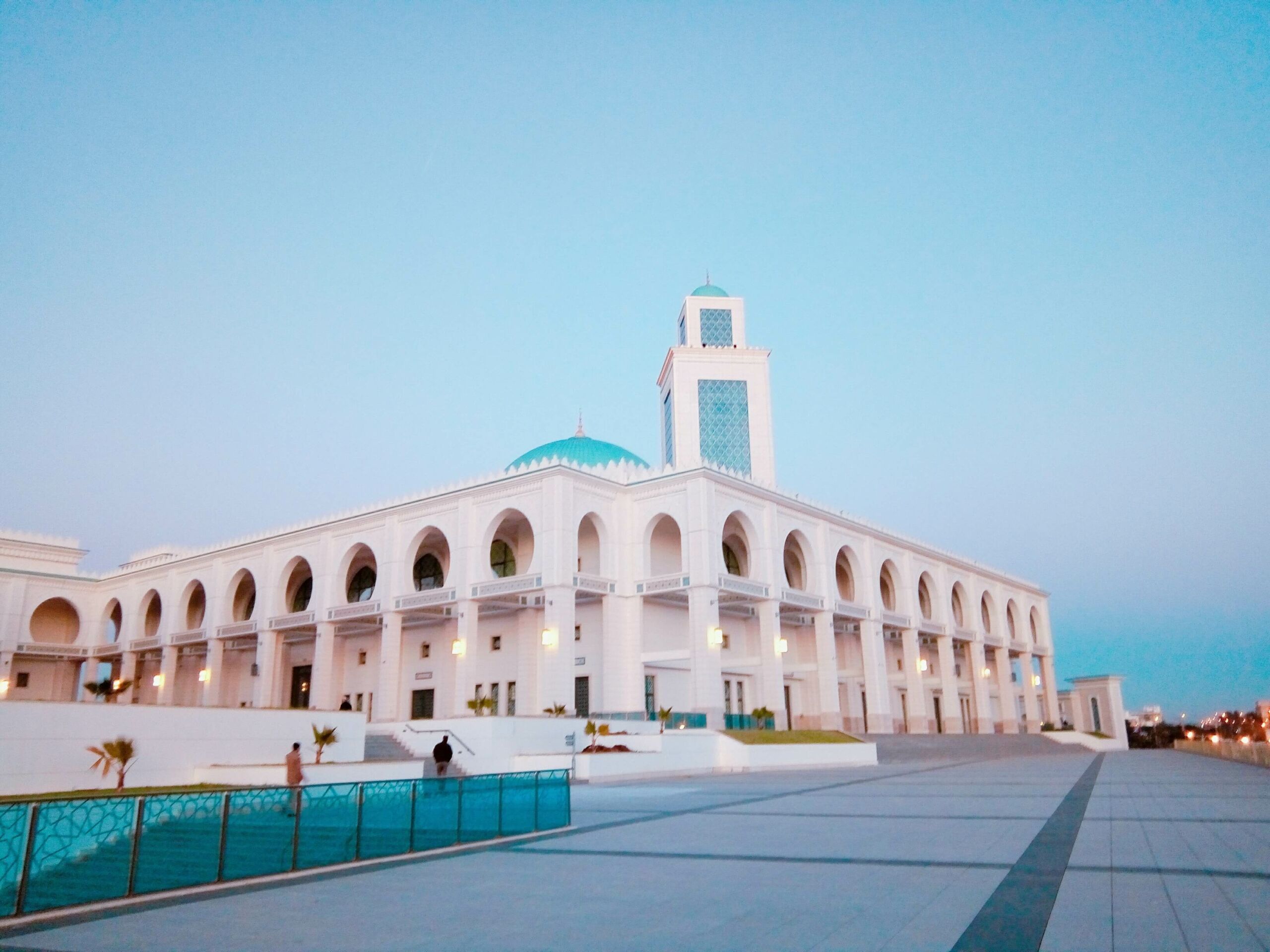
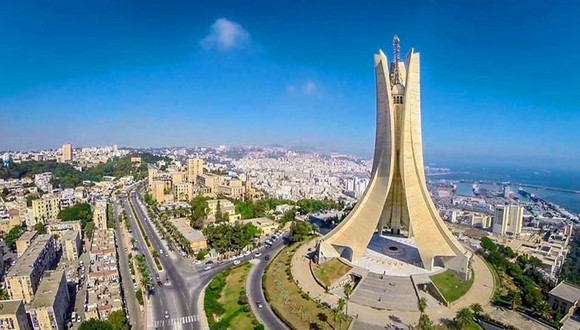
align-items: flex-end
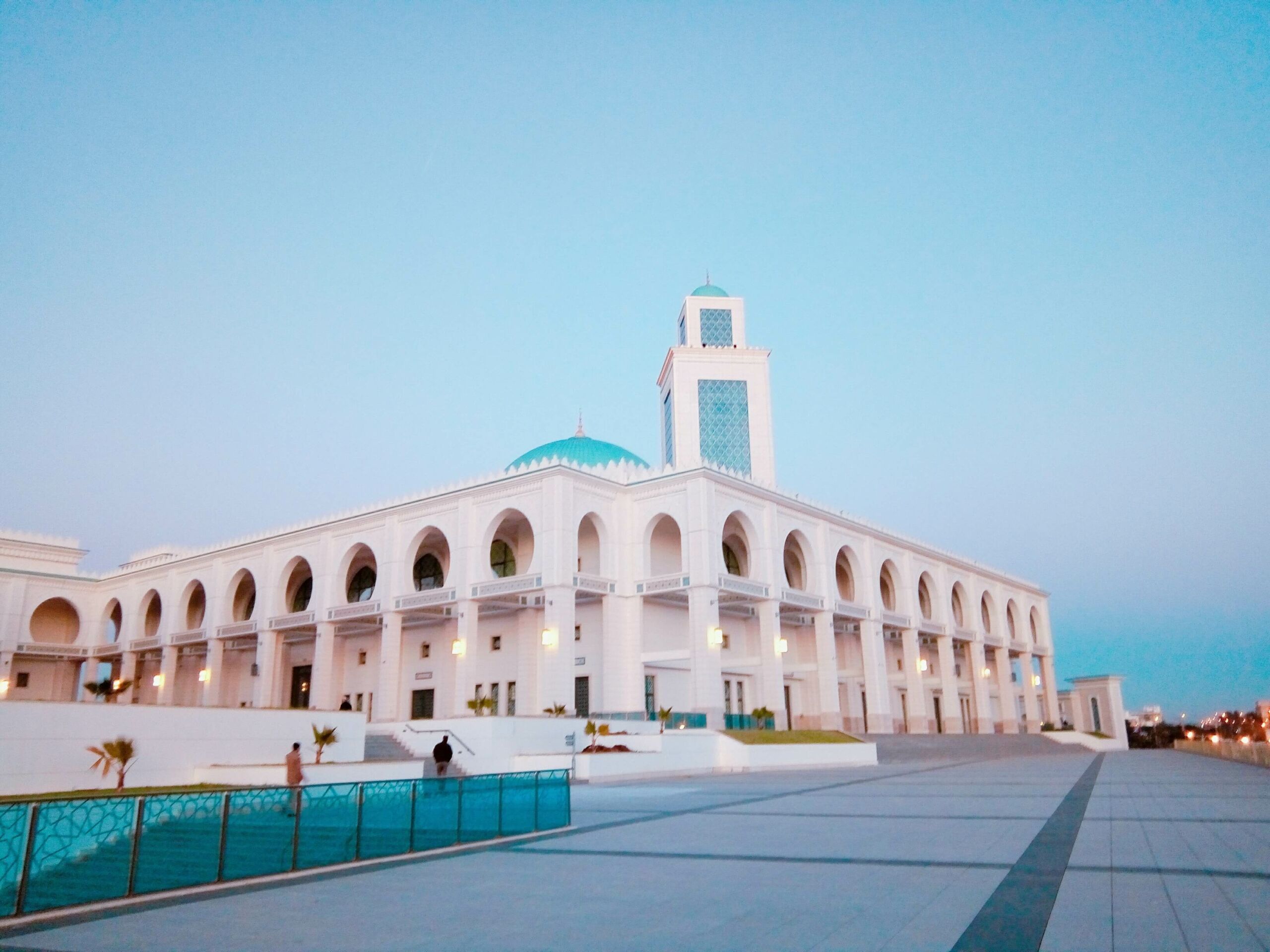
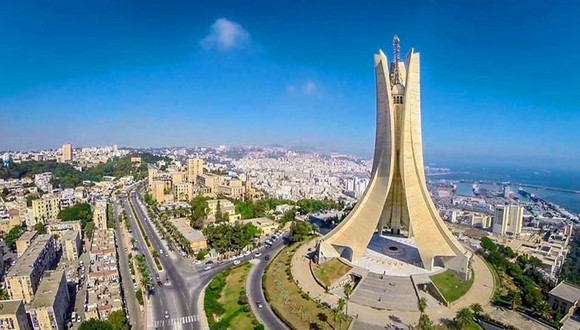
align-items: center
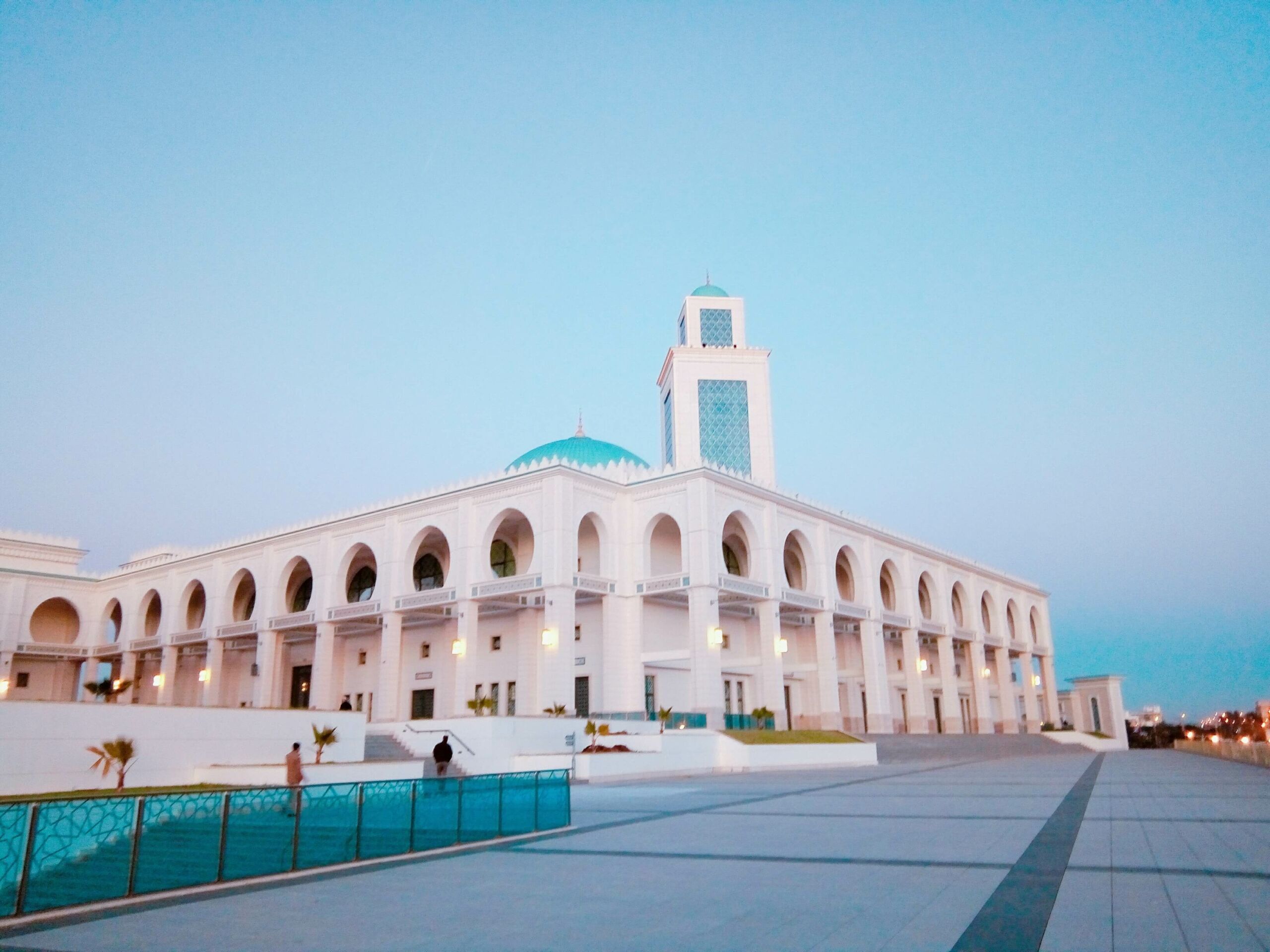
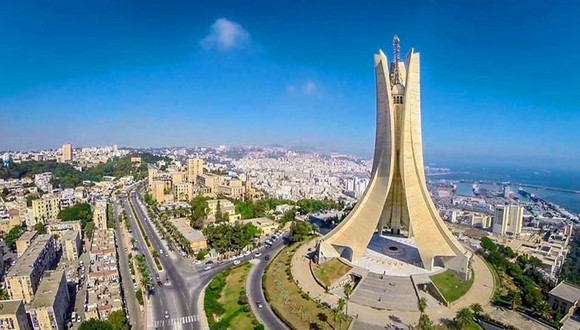
align-items: stretch
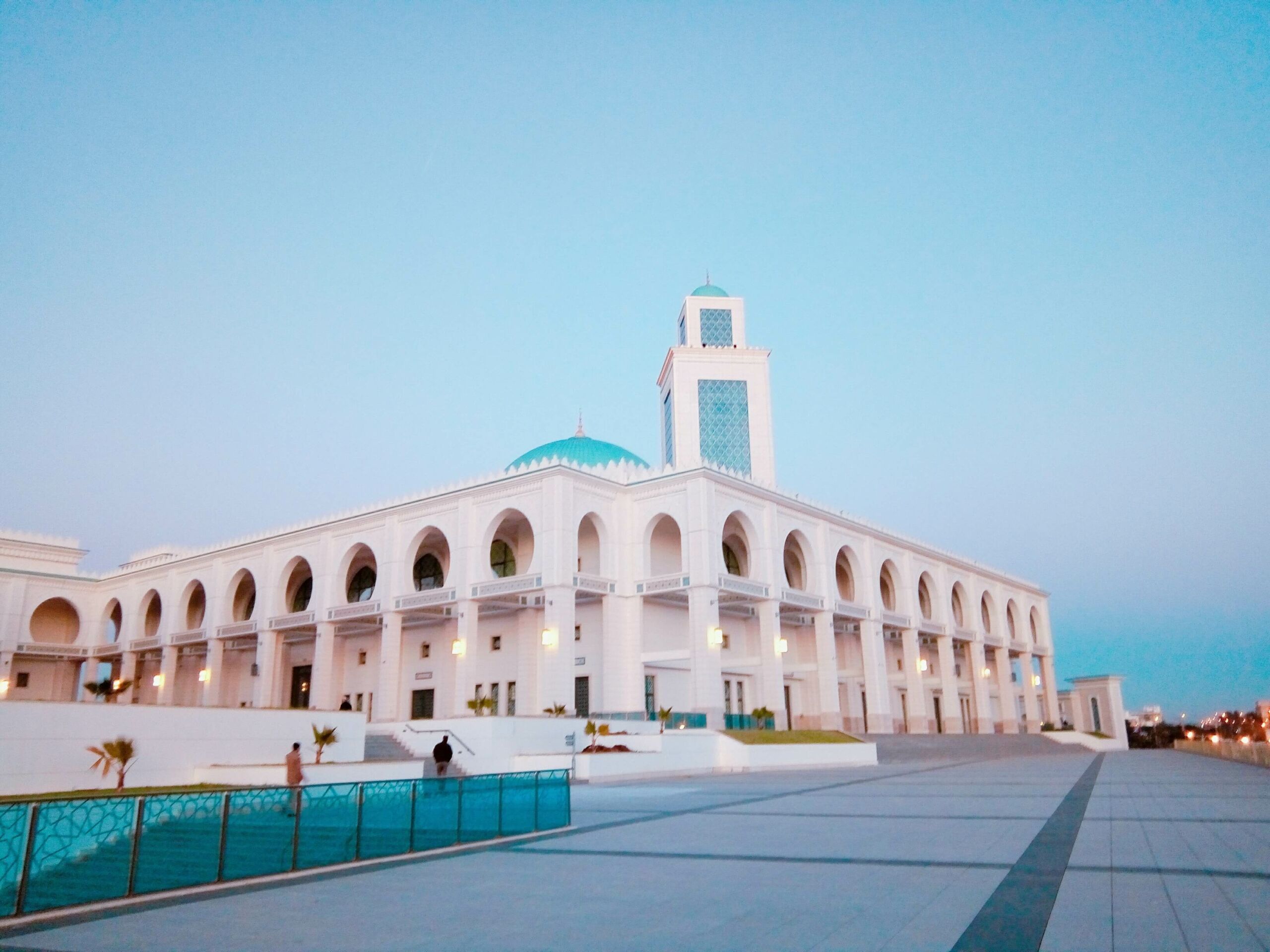
align-items: baseline
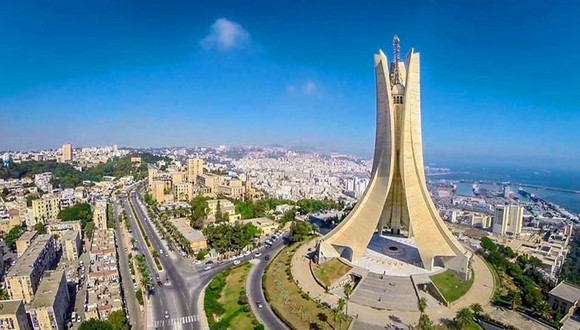
align-content
If flex-wrap is enabled, this controls the spacing of the flex rows along the cross axis. If items don't wrap, this property is ignored.
HTML
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
display: flex;
align-content: flex-start;
}
Result
align-content: flex-start
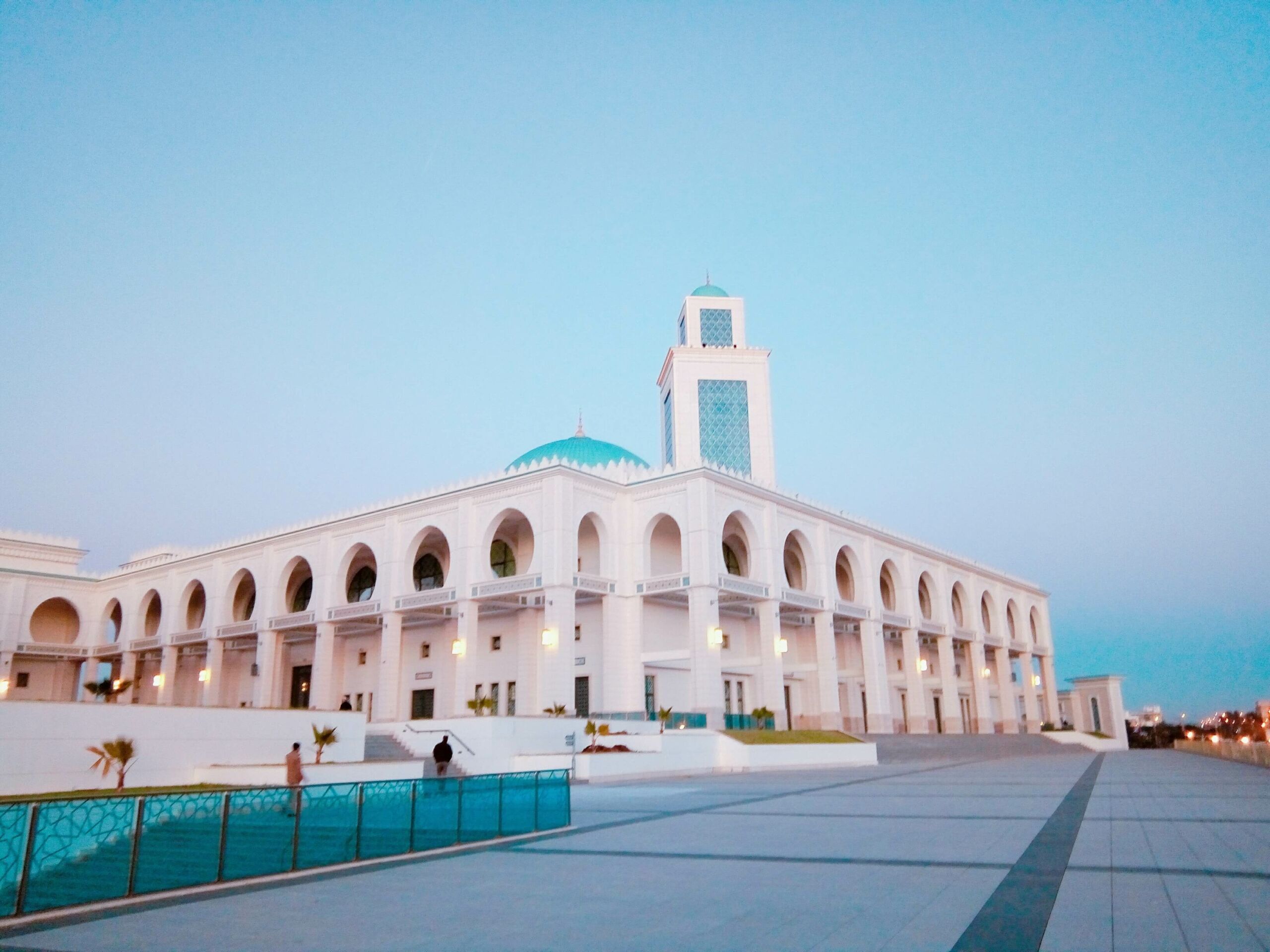
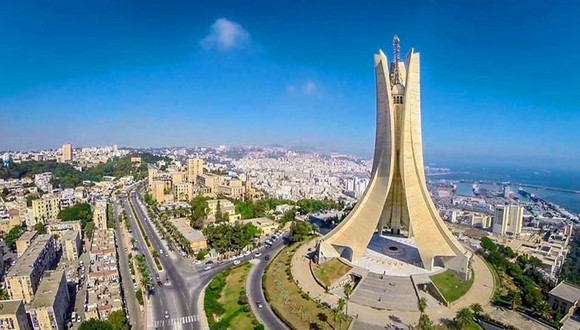
align-content: flex-end
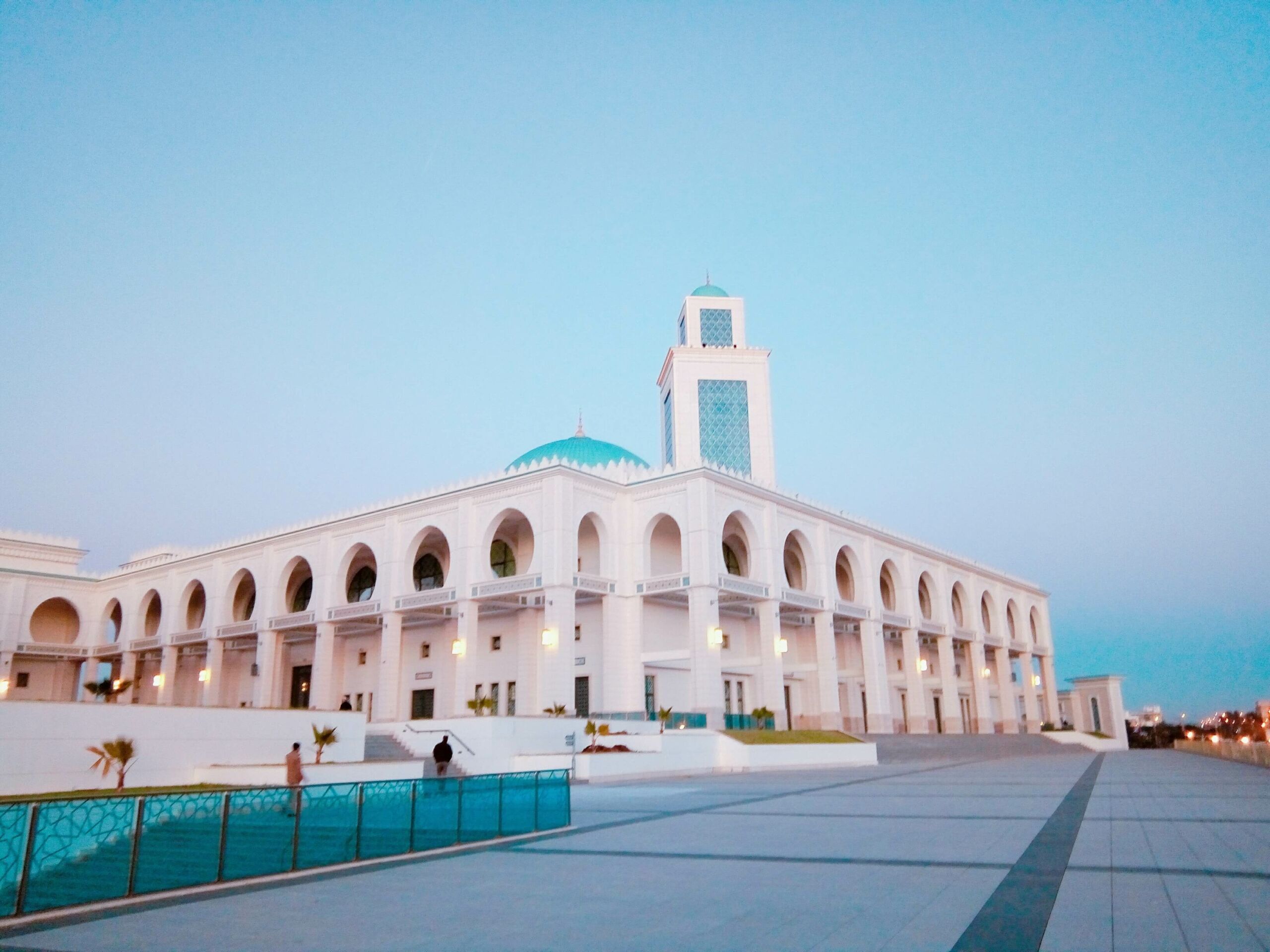
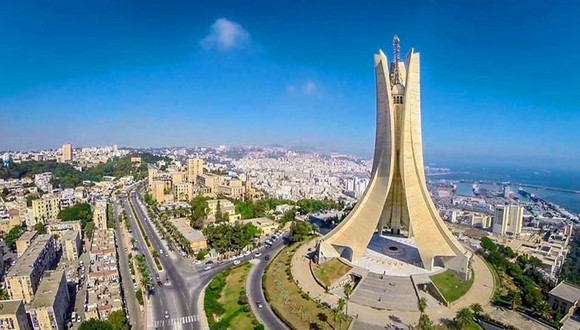
align-content: center
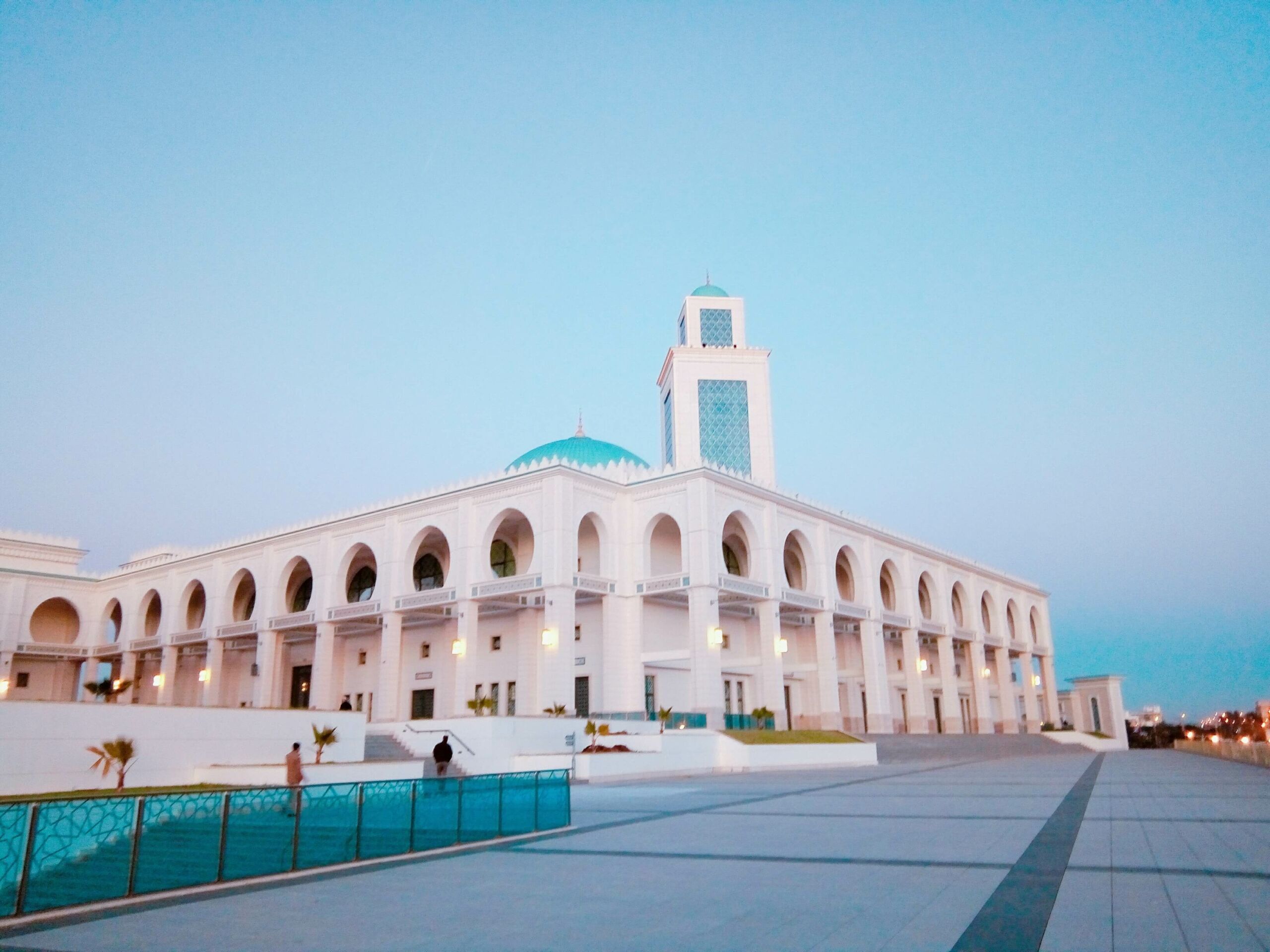
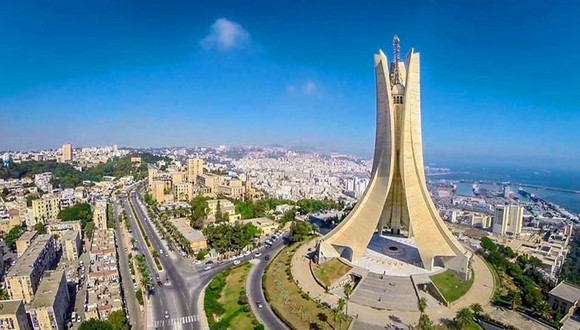
align-content: stretch
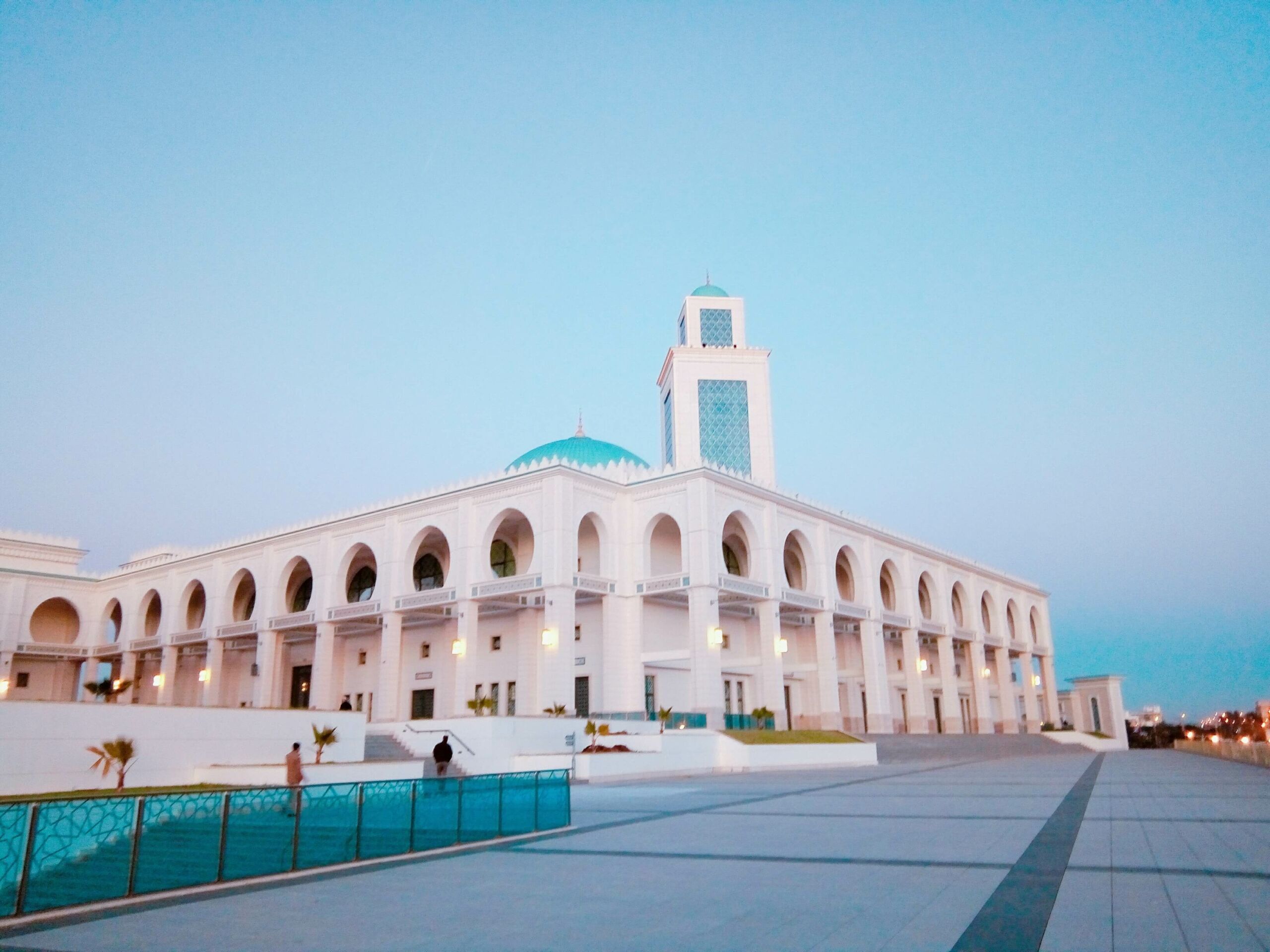
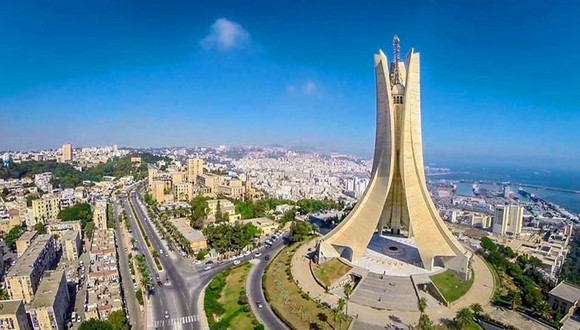
Order
order An integer that moves a flex item to a specific position among its siblings, disregarding source order. Initially, all flex items have an order of 0. Specifying a value of -1 to one item will move it to the beginning of the list, and a value of 1 will move it to the end. You can specify order values for each item to rearrange them however you wish. The numbers don't necessarily need to be consecutive.
HTML
CSS
order: 1;
}
Result
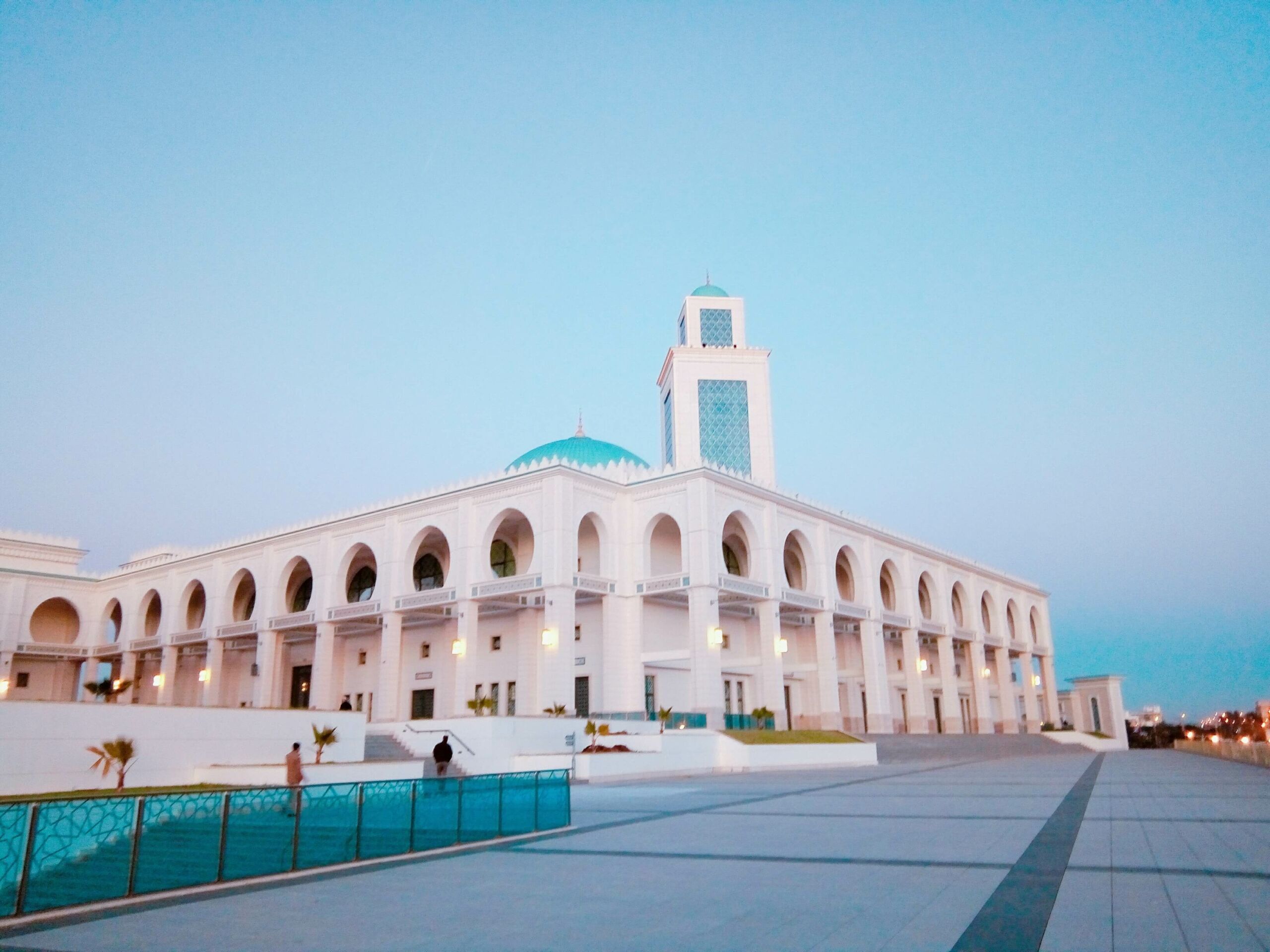
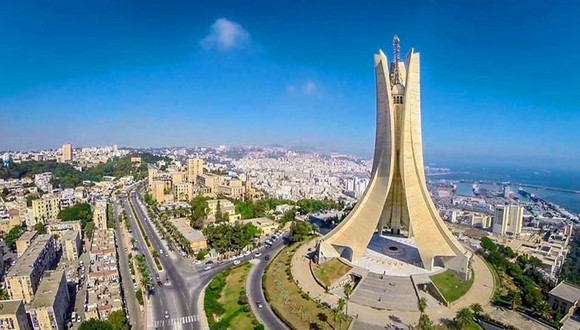
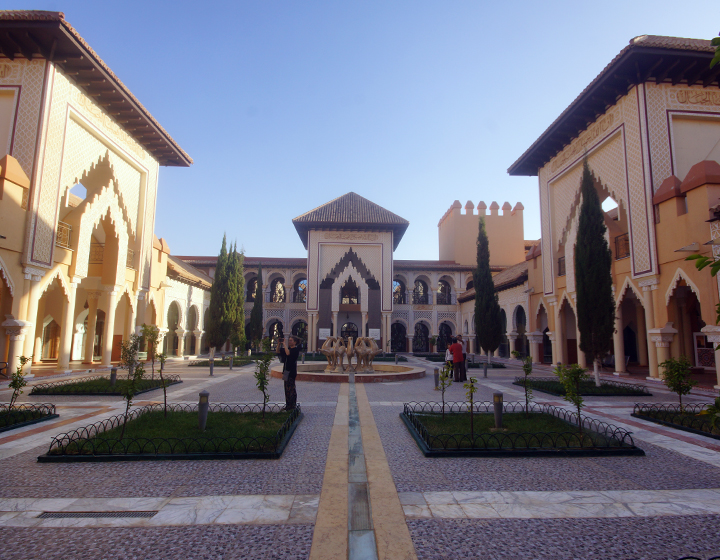
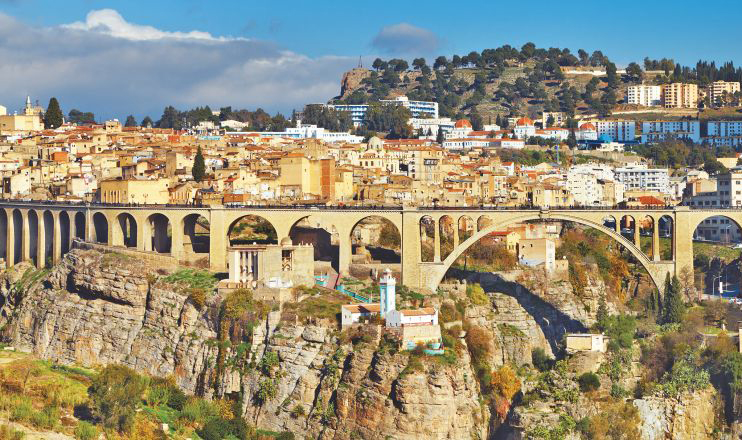
Grid
Grid lets you define a two-dimensional layout of columns and rows and then place elements within the grid. An element with display: grid becomes a grid container. Its child elements then become grid items. Use grid-template-columns and grid-template-rows to define the size of each of the columns and rows in the grid.
HTML
< div > < /div >
< div > < /div >
< div > < /div >
< /div >
CSS
display:grid;
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: 1fr 1fr;
}
Result
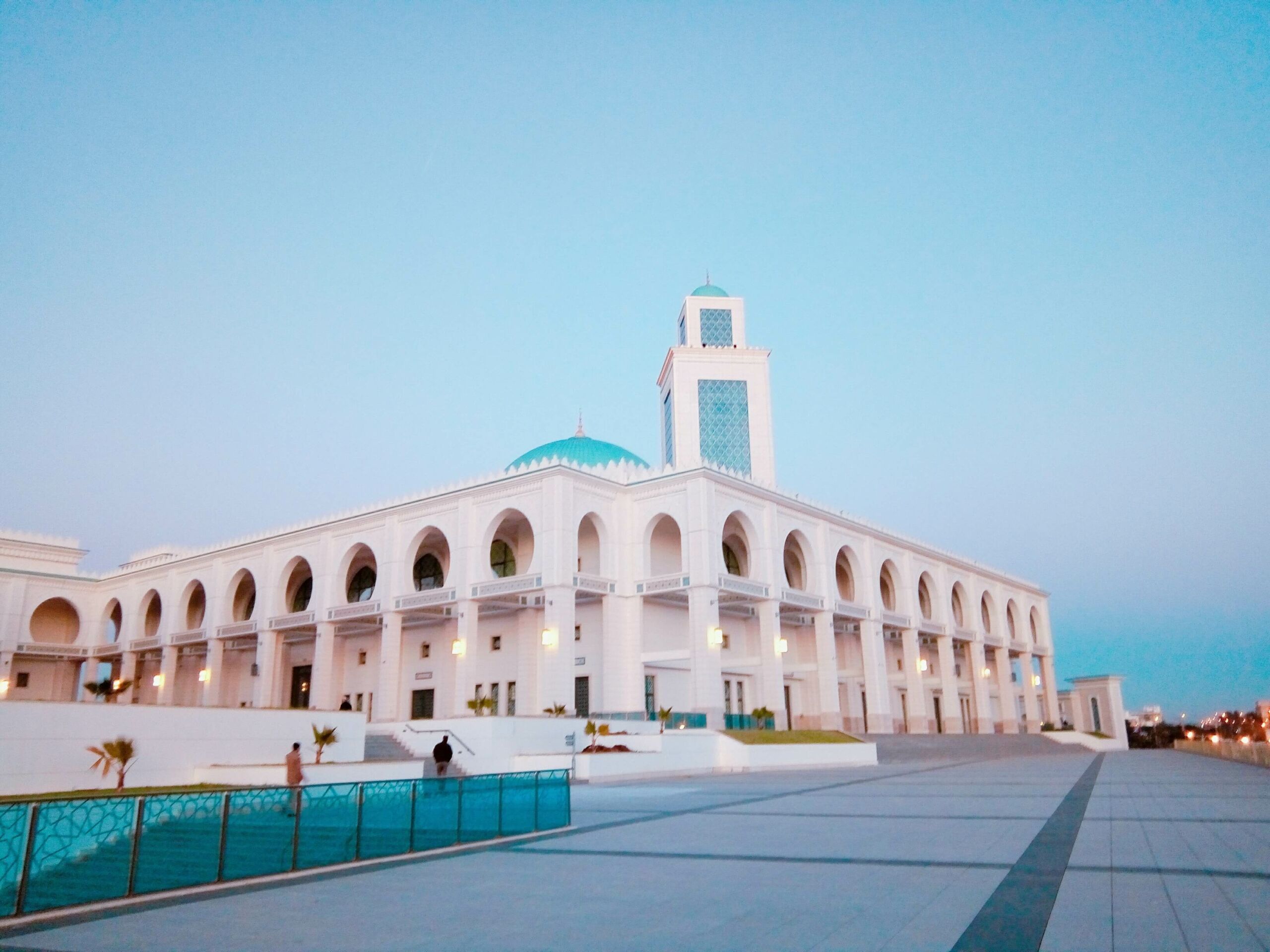
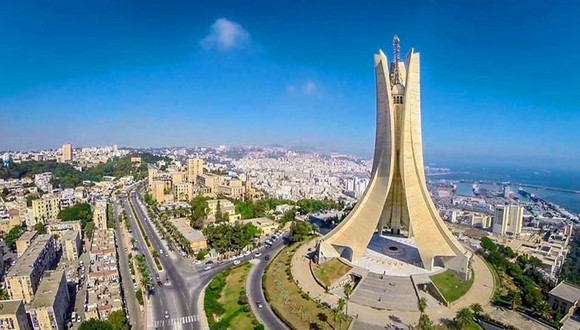
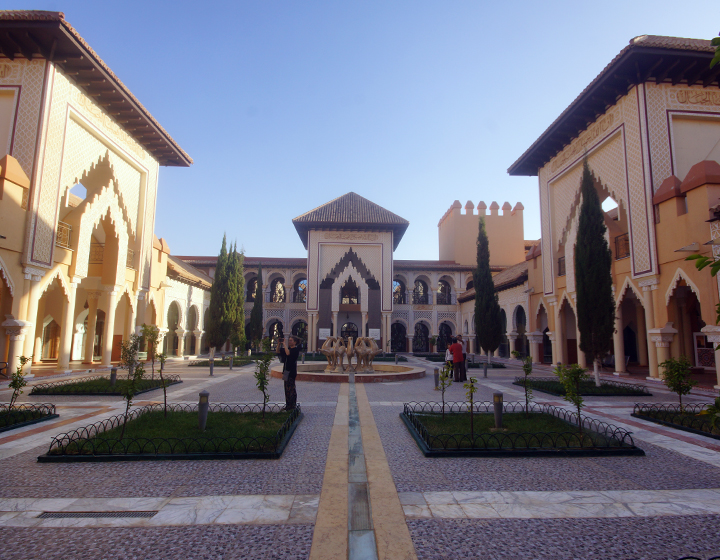
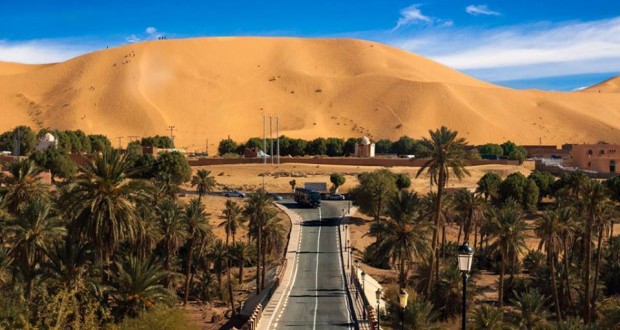
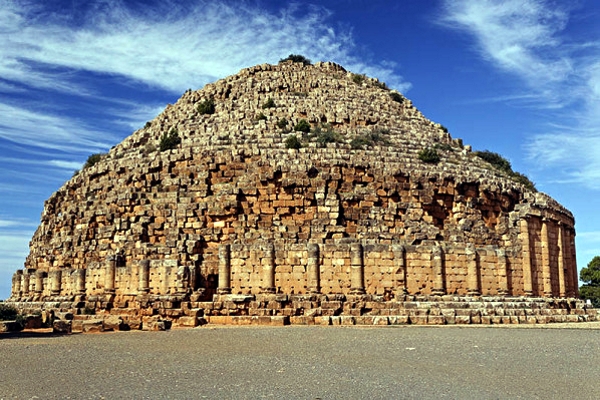
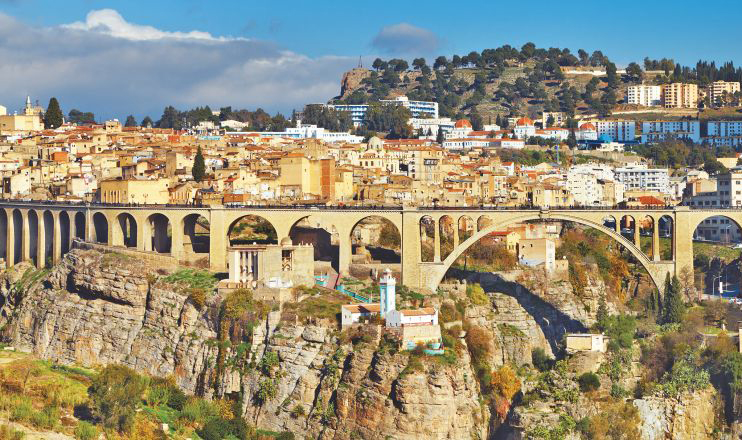
HTML
< div > < /div >
< div > < /div >
< div > < /div >
< /div >
CSS
display:grid;
grid-template-columns: 2fr 1fr;
grid-template-rows: 1fr 1fr;
}
Result
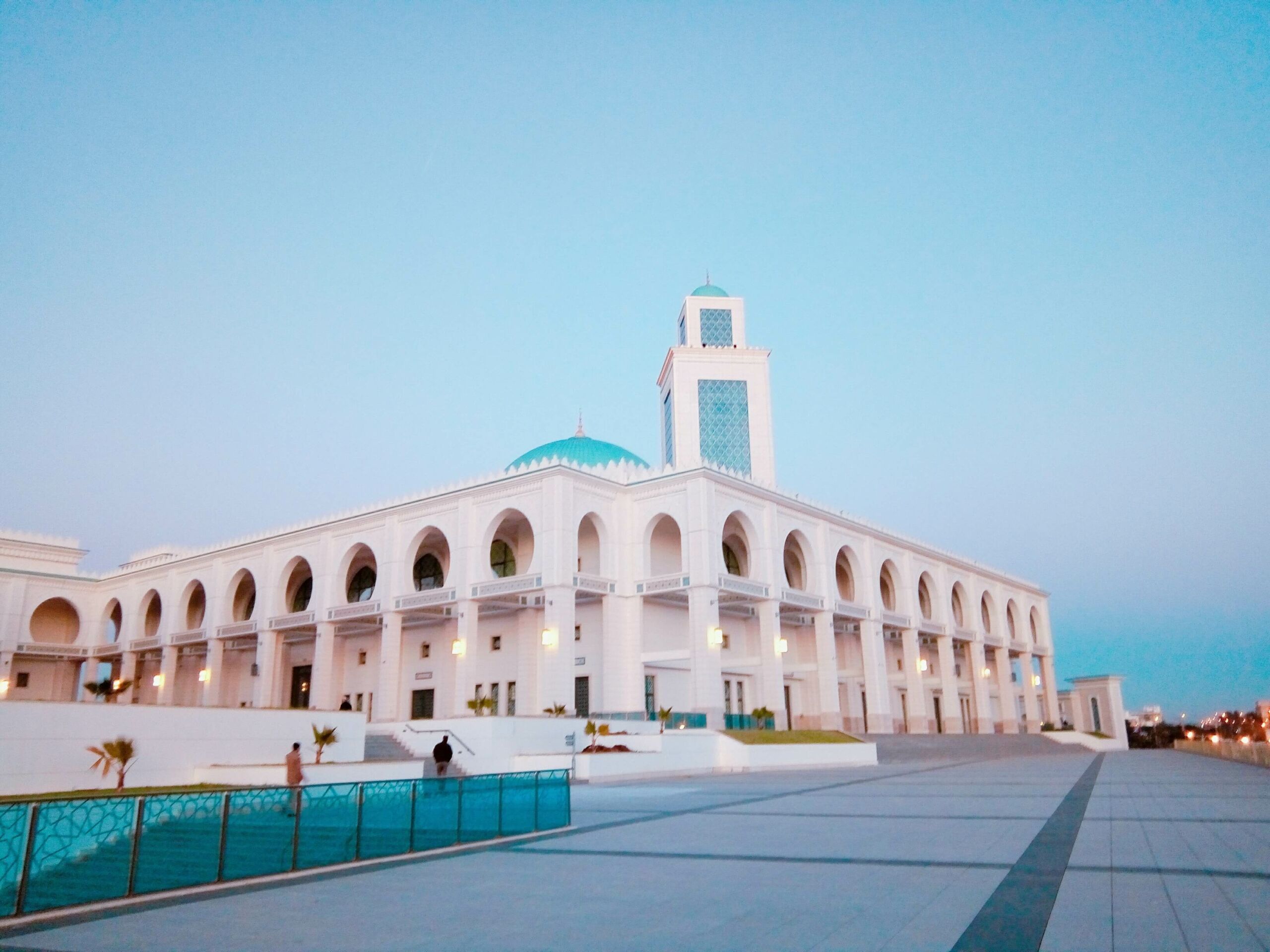
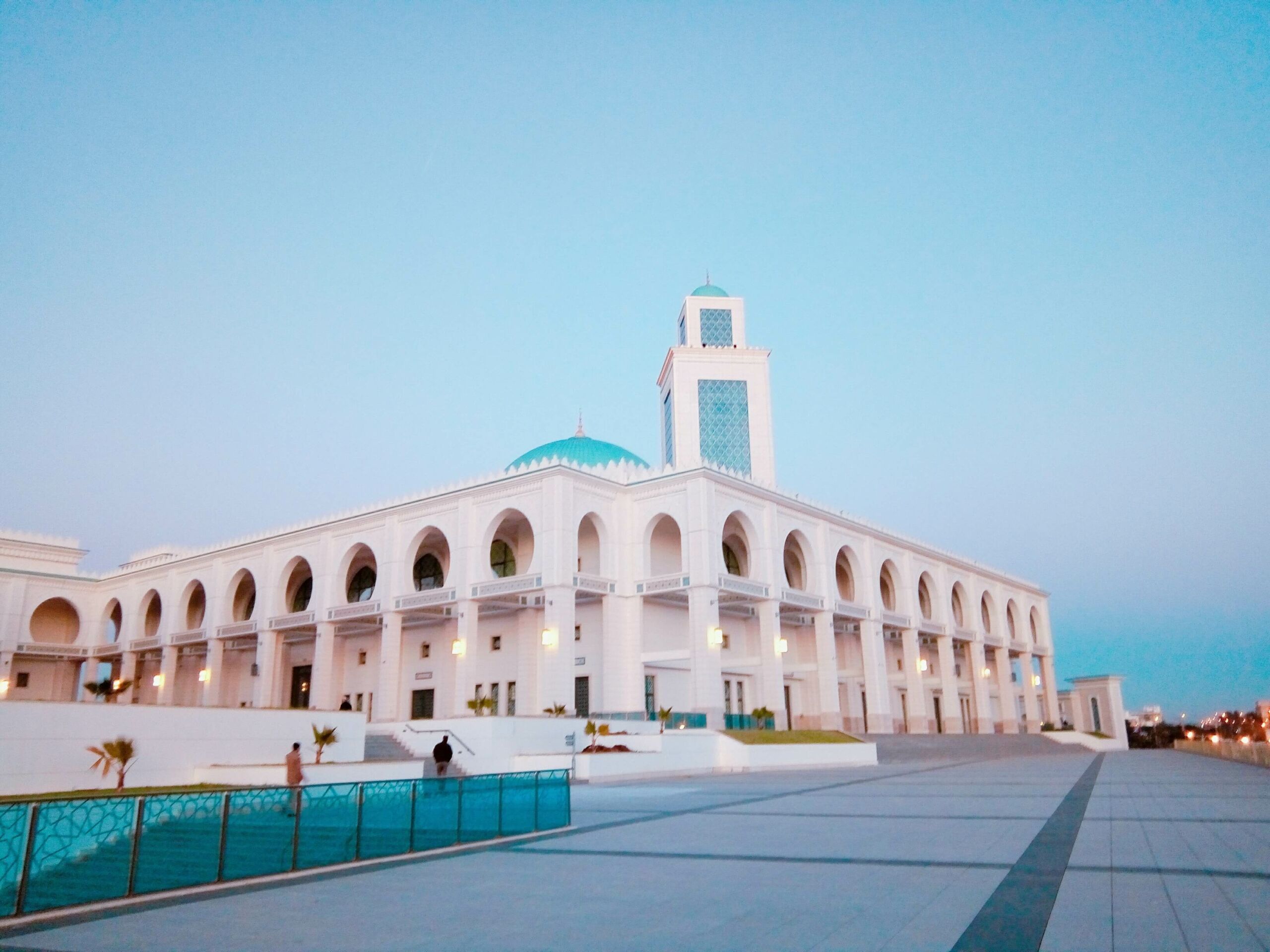
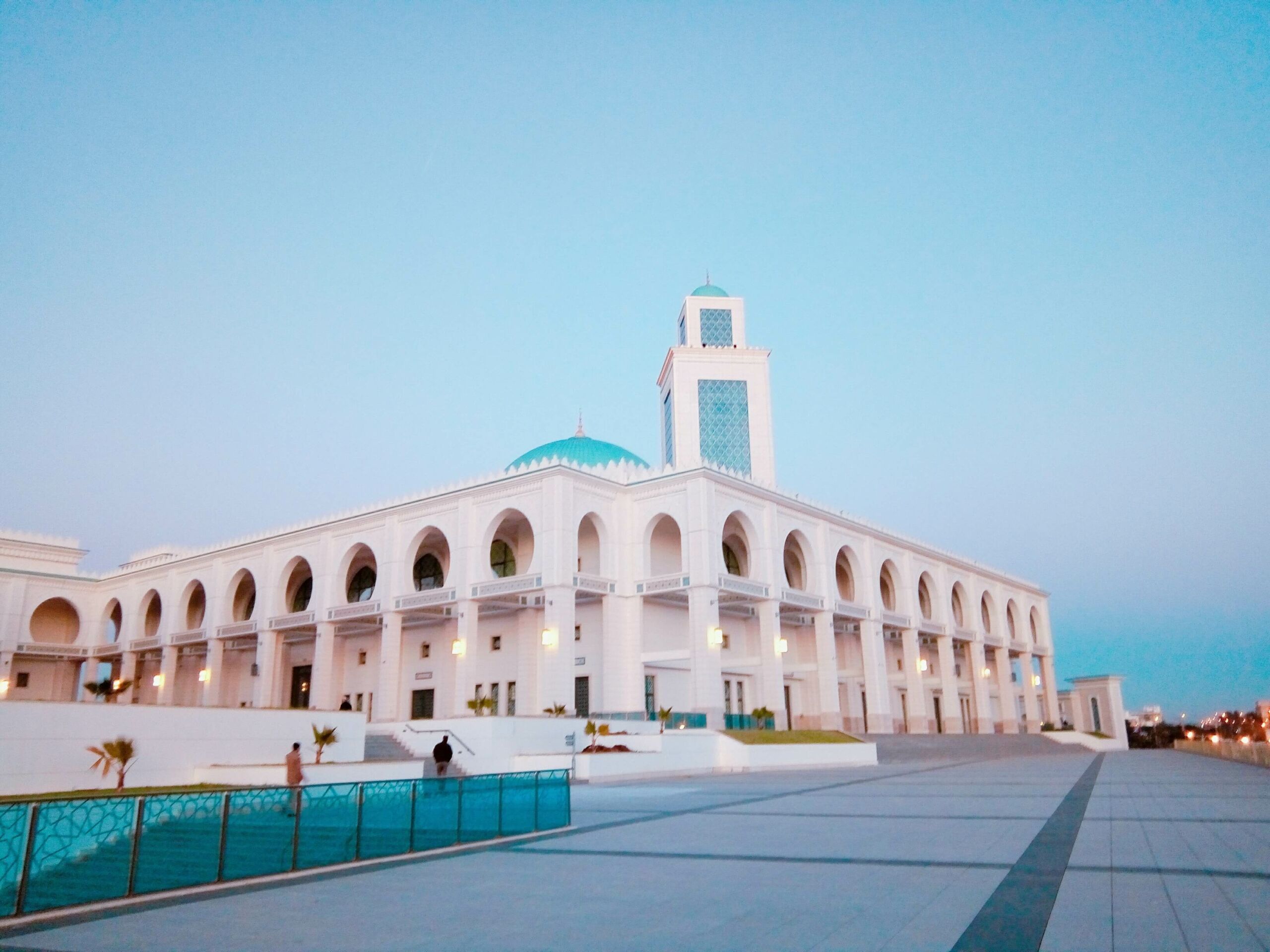
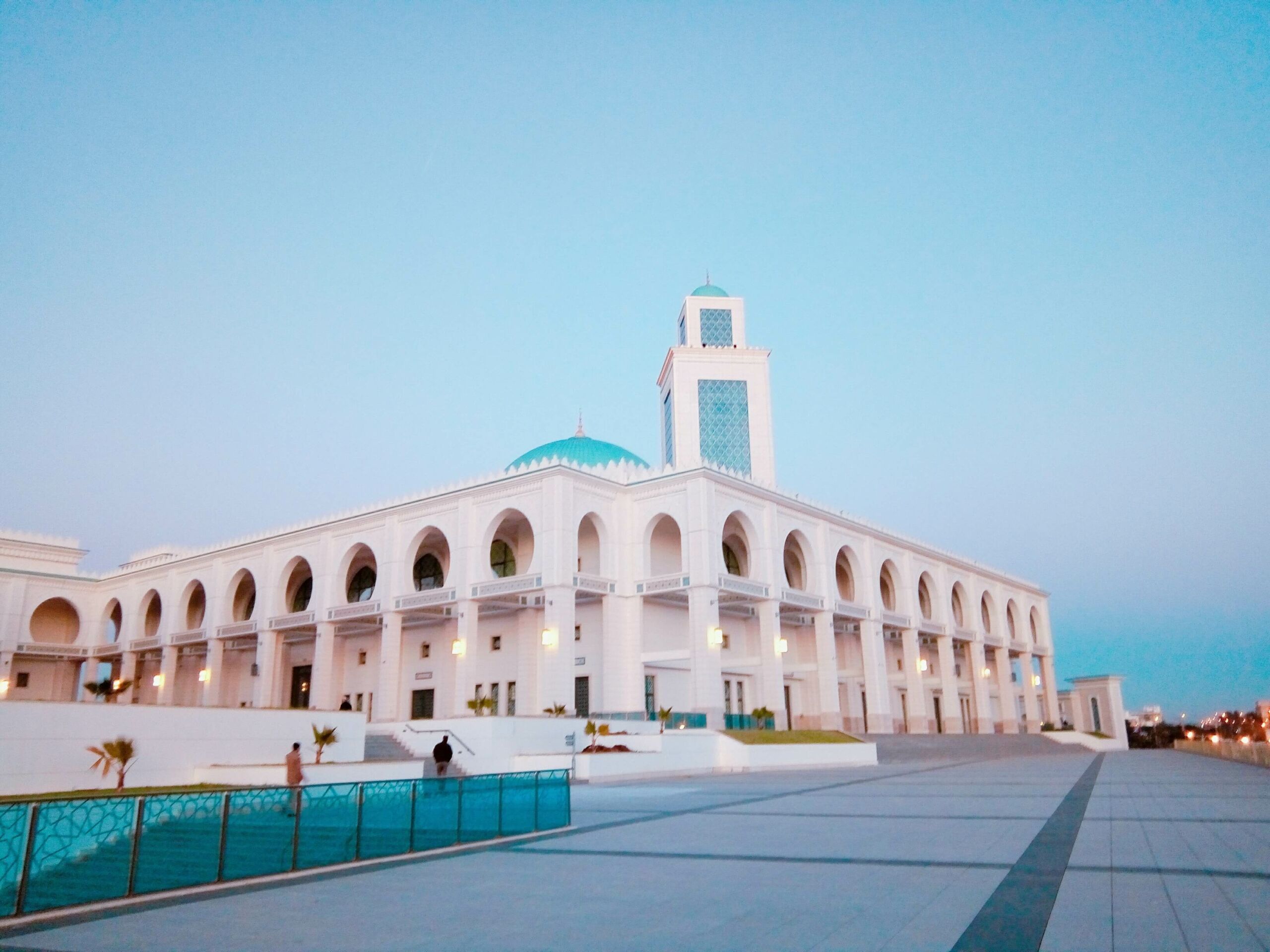
The position Property
The position property specifies the type of positioning method used for an element.
There are five different position values:
- static: are not affected by the top, bottom, left, and right properties.
- relative: the top, right, bottom, and left properties of a relatively-positioned element will cause it to be adjusted away from its normal position.
- fixed: positioned relative to the viewport, which means it always stays in the same place even if the page is scrolled.
- absolute: is positioned relative to the nearest positioned ancestor
- sticky: is positioned based on the user's scroll position.