Unit Testing
Composer: A Dependency Manager for PHP
Composer is a tool for dependency management in PHP. It allows you to
declare the libraries your project depends on and it will manage
(install/update) them for you.
Composer is not a package manager in the same sense as Yum or Apt are.
Yes, it deals with "packages" or libraries, but it manages them on a
per-project basis, installing them in a directory (e.g. vendor) inside
your project. By default it will never install anything globally.
Moreover, Composer supports autoloading of classes, which means that
you can use classes without even including the files that define them.
How to install Composer?
Composer offers a convenient installer that you can execute directly
from the command line. Feel free to download this file or review it on
GitHub if you wish to know more about the inner workings of the
installer.
To install composer, run the following command in your terminal:
php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');"
php -r "if (hash_file('sha384', 'composer-setup.php') === 'e0012edf3e80b6978849f5eff0d4b4e4c79ff1609dd1e613307e16318854d24ae64f26d17af3ef0bf7cfb710ca74755a') { echo 'Installer verified'; } else { echo
'Installer corrupt'; unlink('composer-setup.php'); } echo PHP_EOL;"
php composer-setup.php
php -r "unlink('composer-setup.php');"
This installer script will simply check some php.ini settings, warn you
if they are set incorrectly, and then download the latest composer.phar
in the current directory. The 4 lines above will, in order:
1. Download the installer to the current directory
2. Verify the installer SHA-384 which you can also cross-check here
3. Run the installer
4. Remove the installer
You can install Composer to a specific directory by using the --install-dir option and providing a target
directory.
php composer-setup.php --install-dir=bin
Alternatively, you can install Composer globally.
php composer-setup.php --install-dir=bin --filename=composer
Note: If the above fails due to permissions, you may need to run it
again with sudo.
Note: Composer requires PHP 5.3.2+ to run. A few sensitive php settings
and compile flags are also required, but when using the installer you
will be warned about any incompatibilities.
Note: If you are running OS X, you can also install Composer by
following the instructions here.
Note: If you are on Windows and have XAMPP installed, you may need to
use the php
command instead of php
when following the
command-line installation instructions above.
Note: If the above fails, you can manually download the installer
here and run the same
commands without the first line.
Note: Composer will just check a few PHP settings and then download
composer.phar to your working directory. This file is the Composer
binary. It is a PHAR (PHP archive), which is an archive format for PHP
which can be run on the command line, amongst other things.
Note: You can place the Composer PHAR anywhere you wish. If you put it
in a directory that is part of your PATH, you can access it globally.
On unixy systems you can even make it executable and invoke it without
directly using the php interpreter.
Note: If you want to run Composer from anywhere on your system, you can
create a global composer
command (on Unix systems) with the
following commands:
curl -sS https://getcomposer.org/installer | php
sudo mv composer.phar /usr/local/bin/composer
Note: If the above fails due to permissions, you may need to run it
again with sudo.
Note: If you prefer a graphical user interface (GUI) instead of the
command line, you can try Composer GUI.
Note: If you want to install Composer to be used by all users on your
system, you may place it in /usr/local/bin
.
Note: If you are using SELinux, make sure you have set the
httpd_execmem
permission to allow PHP to execute external
programs, otherwise you will get a cryptic error message like
Failed to execute the Phar
or phar error: exec
failed
.
Note: If you are using cPanel, you will need to use the command line
method listed above.
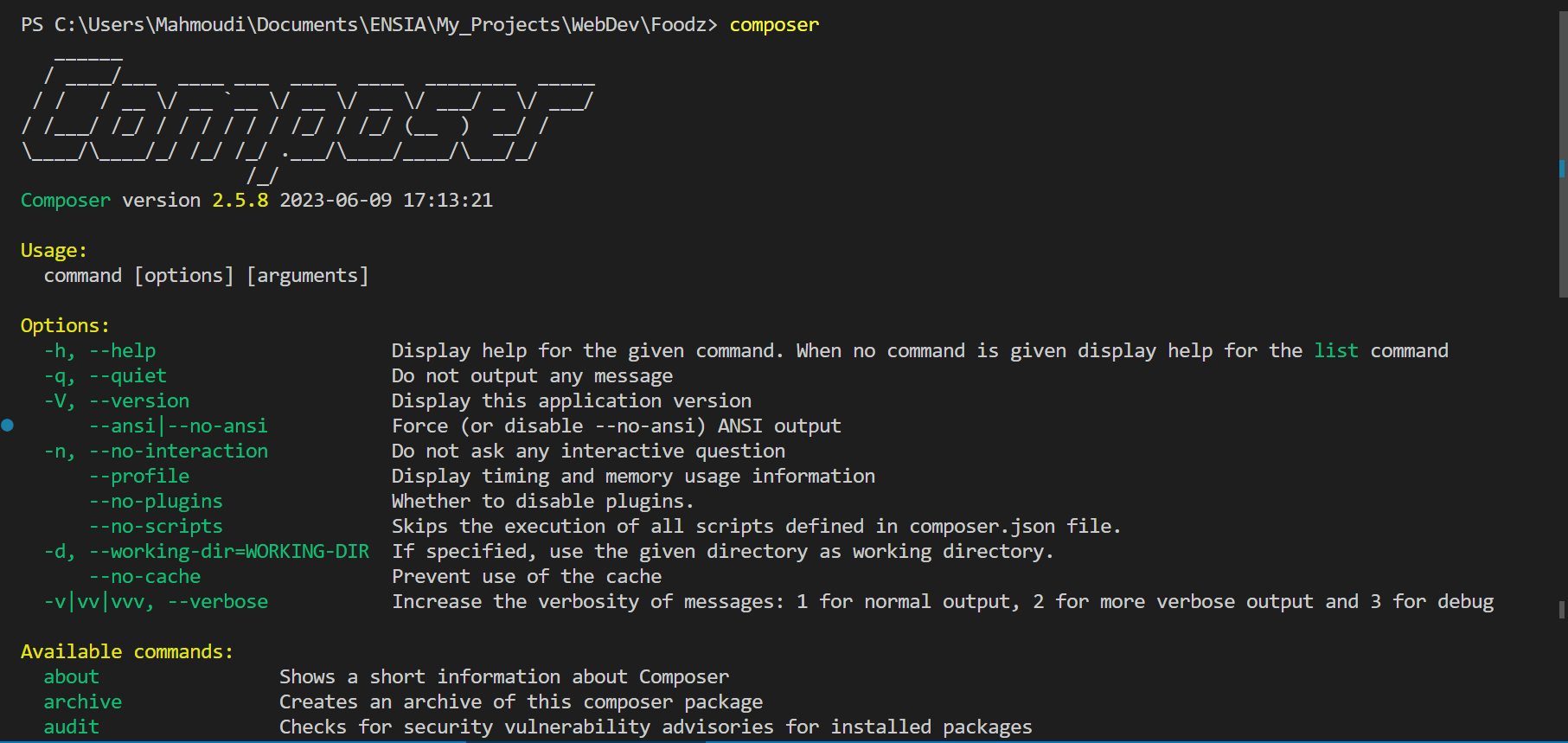
PHPUnit: The PHP Testing Framework
PHPUnit is a programmer-oriented testing framework for PHP. It is an
instance of the xUnit architecture for unit testing frameworks.
PHPUnit is the de-facto standard for unit testing in PHP projects. It
provides both a framework that makes the writing of tests easy as well as
the functionality to easily run the tests and analyse their results.
PHPUnit is distributed through PHAR
and available on Packagist.
It can be installed globally in seconds and is then available to check
your application.
PHPUnit 9.0 supports PHP 7.3, PHP 7.4, and PHP 8.0.
PHPUnit 8.5 supports PHP 7.2, PHP 7.3, and PHP 7.4.
PHPUnit 7.5 supports PHP 7.1, PHP 7.2, and PHP 7.3.
PHPUnit 6.5 supports PHP 7.0, PHP 7.1, and PHP 7.2.
PHPUnit 5.7 supports PHP 5.6, PHP 7.0, and PHP 7.1.
PHPUnit installation with Composer
The recommended way to install PHPUnit is via Composer.
composer require --dev phpunit/phpunit
Composer will install the proper PHPUnit version in the vendor/bin
directory.
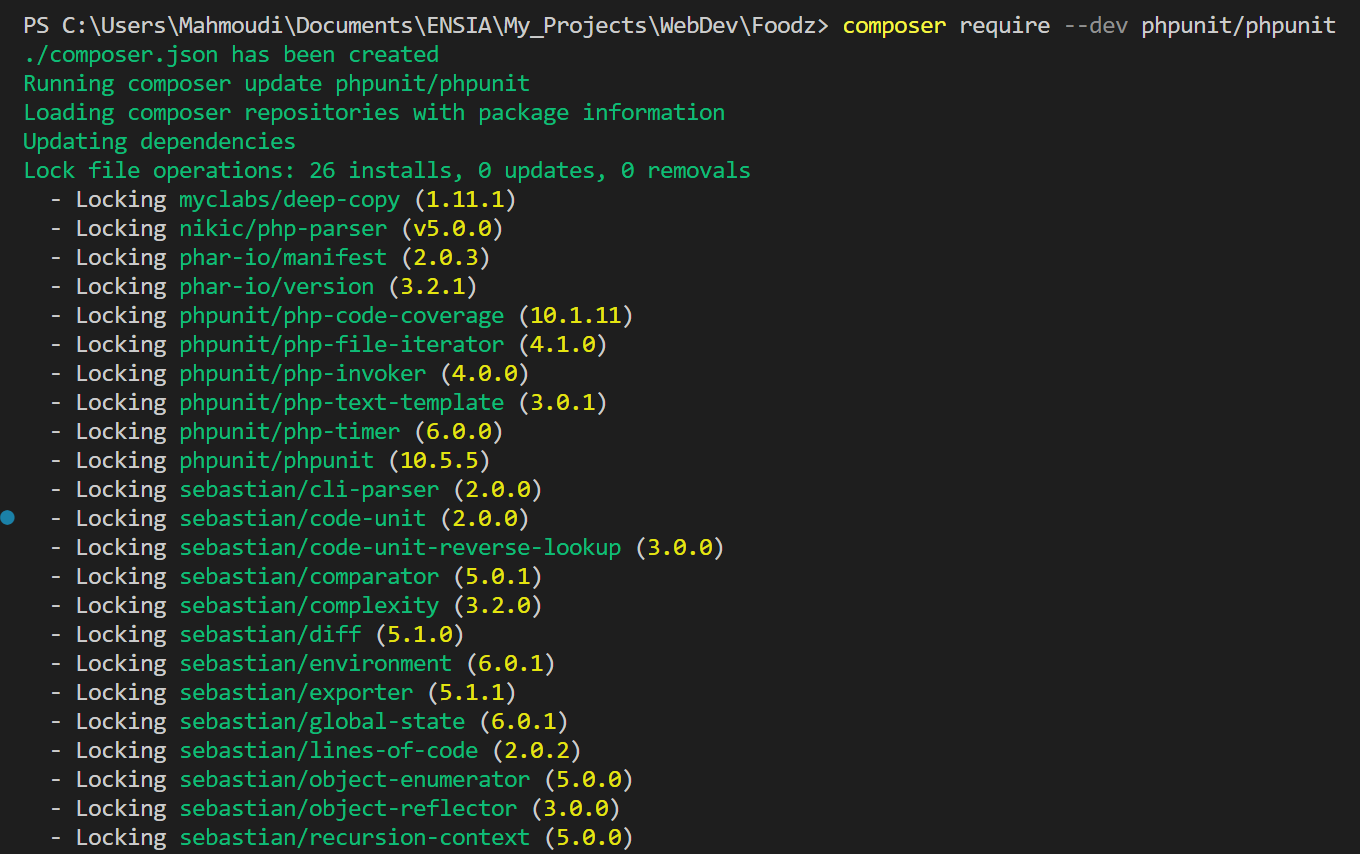
To verify that PHPUnit has been installed correctly, run the
phpunit --version
command.
