Sessions, Cookies, and Authentification
- HTTP is a stateless protocol, which means that each request is independent of the others. This is
fine for most web pages, but it can be a problem if you want to keep track of users as they move
around your site. For example, you might want to keep track of users so that you can:
- Personalize the content of your site for them
- Keep track of their shopping carts
- Keep track of their progress through a multi-page form
- Keep track of their login status
- PHP provides three ways to keep track of users:
- Using cookies
- Using sessions
- Using HTTP authentication
Cookies
- A cookie is an item of data that a web server saves to your computer's hard disk via a web browser.
- It can contain almost any alphanumeric information (as long as it's under 4 KB) and can be retrieved from your computer and returned to the server
- Because of their privacy implications, cookies can be read only from the issuing domain.
- Third-party cookies are cookies that are set by a domain other than the one you are currently visiting. For example, if you visit a site that has a Facebook Like button, Facebook will set a cookie on your computer. This cookie will be sent to Facebook when you visit other sites that have Facebook Like buttons. This allows Facebook to track your browsing habits across the web.
- Cookies are exchanged during the transfer of headers, before the actual HTML of a web page is sent, and it is impossible to send a cookie once any HTML has been transferred.
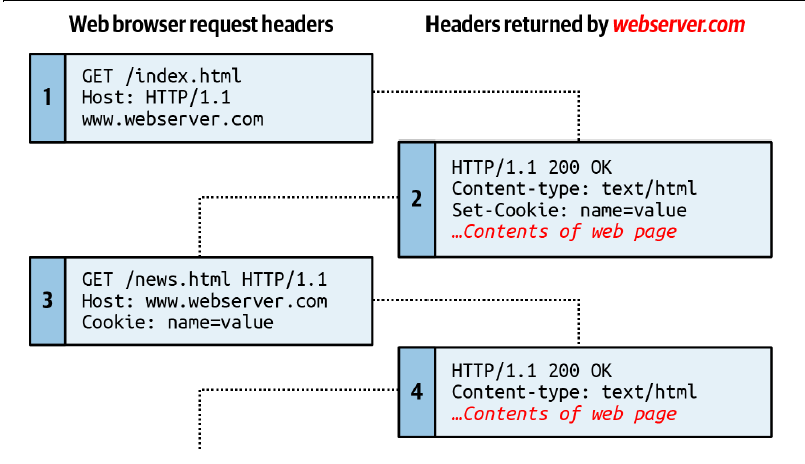
This exchange shows a browser receiving two pages:
- The browser issues a request to retrieve the main page, index.html, at the website http://www.webserver.com. The first header specifies the file, and the second header specifies the server.
- When the web server at webserver.com receives this pair of headers, it returns some of its own. The second header defines the type of content to be sent (text/ html), and the third one sends a cookie of the name name and with the value value. Only then are the contents of the web page transferred.
- Once the browser has received the cookie, it will then return it with every future request made to the issuing server until the cookie expires or is deleted. So, when the browser requests the new page /news.html, it also returns the cookie name with the value value.
- Because the cookie has already been set, when the server receives the request to send /news.html, it does not have to resend the cookie but just returns the requested page.
Setting a Cookie
setcookie(name, value, expire, path, domain, secure, httponly);
Parameter | Description | Example |
name | The name of the cookie. This is the name that your server will use to access the cookie on subsequent browser requests. | location |
value | The value of the cookie, or the cookie's contents. This can contain up to 4 KB of alphanumeric text. | Algeria |
expire | (Optional.) The Unix timestamp of the expiration date. Generally, you will probably use time() plus a number of seconds. If not set, the cookie expires when the browser closes. | time() + 2592000 |
path | (Optional.) The path of the cookie on the server. If this is a / (forward slash), the cookie is available over the entire domain, such as www.webserver.com. If it is a subdirectory, the cookie is available only within that subdirectory. The default is the current directory that the cookie is being set in, and this is the setting you will normally use. | / |
domain | (Optional.) The internet domain of the cookie. If this is webserver.com, the cookie is available to all of webserver.com and its subdomains, such as www.webserver.com and images.webserver.com. If it is images.webserver.com, the cookie is available only to images.webserver.com and its subdomains, such as sub.images.webserver.com, but not, say, to www.webserver.com. | webserver.com |
secure | (Optional.) Whether the cookie must use a secure connection (https://). If this value is TRUE, the cookie can be transferred only across a secure connection. The default is FALSE. | FALSE |
httponly | (Optional; implemented since PHP version 5.2.0.) Whether the cookie must use the HTTP protocol. If this value is TRUE, scripting languages such as JavaScript cannot access the cookie. The default is FALSE. | FALSE |
PHP
<?php
setcookie('location', 'Algeria', time() + 60 * 60 * 24 * 7, '/');
?>
setcookie('location', 'Algeria', time() + 60 * 60 * 24 * 7, '/');
?>
Result
Create a cookie with the name location and the value Algeria that is accessible across the entire web server on the current domain, and will be removed from the browser's cache in seven days
Accessing a Cookie
- Reading the value of a cookie is as simple as accessing the $_COOKIE system array.
- Note that you can read a cookie back only after it has been sent to a web browser. This means that when you issue a cookie, you cannot read it in again until the browser reloads the page (or another with access to the cookie) from your website and passes the cookie back to the server in the process.
PHP
<?php
if (isset($_COOKIE['location']))
$location = $_COOKIE['location'];
?>
if (isset($_COOKIE['location']))
$location = $_COOKIE['location'];
?>
Result
Checks if the current browser has the cookie called location already stored and, if so, to read its value.
Destroying a Cookie
- To destroy a cookie, you must set it to a date in the past. This will cause the browser to delete the cookie.
- It is important to note that you cannot destroy a cookie by unsetting it from the $_COOKIE array. This will simply remove the cookie from the array, but the browser will still have it stored.
- It is important for all parameters in your new setcookie call except the timestamp to be identical to the parameters when the cookie was first issued; otherwise, the deletion will fail.
- You may also provide an empty string for the cookie value (or a value of FALSE), and PHP will automatically set its time in the past for you.
PHP
<?php
setcookie('location', '', time() - 3600, '/');
?>
setcookie('location', '', time() - 3600, '/');
?>
Result
Destroy the cookie called location by setting it to a date in the past.
See code example
Sessions
- These are groups of variables that are stored on the server but relate only to the current user.
- To ensure that the right variables are applied to the right users, PHP saves a cookie in the users’ web browsers to uniquely identify them. This cookie is called the session ID.
- This cookie has meaning only to the web server and cannot be used to ascertain any information about a user.
Implementing Simple Sessions
The basic steps of using sessions in PHP are:
- Starting a session
- Registering session variables
- Using session variables
- Deregistering variables and destroying the session
Starting a session
There are two ways you can do this:
- The first, and simplest, is to begin a script with a call to the session_start() function.
This function checks to see whether there is already a current session. If not, it will create one, providing access to the superglobal $_SESSION array. If a session already exists, session_start() loads the registered session variables so that you can use them. -
The second way you can begin a session is to set PHP to start one automatically when someone
comes to your site. You can do this by using the session.auto_start option in your php.ini
file.
This option is set to 0 by default, which means that sessions are not started automatically. If you set it to 1, sessions will be started automatically (See doc).
This is not recommended, however, because it can cause problems if you have a lot of traffic on your site. It is better to start sessions only when you need them.
Note: If you are using a hosting service, you may not have access to the php.ini file. In that case, you will have to use the first method.
PHP
<?php
session_start();
?>
session_start();
?>
Result
This will start a session.
Registering session variables
- Session variables are stored in the superglobal $_SESSION array. To create a session variable, you simply set an element in this array.
- The session variable you have just created will be tracked until the session ends or until you manually unset it. The session may also naturally expire based on the session.gc_maxlifetime setting in the php.ini file. This setting determines the amount of time (in seconds) that a session will last before it is ended by the garbage collector. By default, this is set to 1440 seconds (24 minutes).
PHP
<?php
$_SESSION['myvar'] = "Any value you want";
?>
$_SESSION['myvar'] = "Any value you want";
?>
Result
Using session variables
- To bring session variables into scope so that they can be used, you must first start a session calling session_start(), as previously mentioned. You can then access the variable via the $_SESSION superglobal array—for example, as $_SESSION['myvar'].
- You need to check whether session variables have been set (via, say, isset() or empty()).
PHP
<?php
// Another page
session_start();
if (isset($_SESSION['myvar']))
{
echo $_SESSION['myvar'];
}
?>
// Another page
session_start();
if (isset($_SESSION['myvar']))
{
echo $_SESSION['myvar'];
}
?>
Result
Any value you want
Deregistering variables and destroying the session
- When you are finished with a session variable, you can unset it. You can do this directly by unsetting the appropriate element of the $_SESSION array.
- You should not try to unset the whole $_SESSION array because doing so will effectively disable sessions.
- When you are finished with a session, you should first unset all the variables and then call session_destroy() to clean up the session ID.
Setting a Timeout
- There are other times when you might wish to close a user's session yourself, such as when the user has forgotten or neglected to log out, and you want the program to do so for them for their own security. You do this by setting the timeout after which a logout will automatically occur if there has been no activity.
-
To do this, use the ini_set function as follows. This example sets the timeout to
exactly one day (the letters gc standing for garbage collection):
ini_set('session.gc_maxlifetime', 60 * 60 * 24); -
If you wish to know what the current timeout period is, you can display it using the following:
echo ini_get('session.gc_maxlifetime');
PHP
<?php
//unset a single variable
unset($_SESSION['myvar']);
/*To unset all the session variables at once, you can clear out the existing elements in the $_SESSION superglobal. */
$_SESSION = array();
//To destroy the session.
session_destroy();
?>
//unset a single variable
unset($_SESSION['myvar']);
/*To unset all the session variables at once, you can clear out the existing elements in the $_SESSION superglobal. */
$_SESSION = array();
//To destroy the session.
session_destroy();
?>
Result
See code example
HTTP Authentication
- HTTP authentication uses the web server to manage users and passwords for the application. It's adequate for simple applications that ask users to log in, although most applications will have specialized needs or more stringent security requirements that call for other techniques.
- To use HTTP authentication, PHP sends a header request asking to start an authentication dialog with the browser. The server must have this feature turned on in order for it to work.
- Basic realm is the name of the section that is protected and appears as part of the pop-up prompt.
- Once a user has been authenticated, you will not be able to get the authentication dialog to pop up again unless the user closes and reopens all browser windows, because the web browser will keep returning the same username and password to PHP.
- HTTP authentication is not secure. The username and password are sent in plain text, so anyone who can intercept the request can read them. You should use HTTP authentication only over a secure connection (https://).
PHP
<?php
$username = 'admin';
$password = '123';
if ( isset($_SERVER['PHP_AUTH_USER']) &&
isset($_SERVER['PHP_AUTH_PW']) ) {
if ( $_SERVER['PHP_AUTH_USER'] === $username &&
$_SERVER['PHP_AUTH_PW'] === $password )
echo "You are now logged in";
else die("Invalid username/password combination");
} else {
header('WWW-Authenticate: Basic realm="Restricted Area"');
header('HTTP/1.0 401 Unauthorized');
die("Please enter your username and password");
}
?>
$username = 'admin';
$password = '123';
if ( isset($_SERVER['PHP_AUTH_USER']) &&
isset($_SERVER['PHP_AUTH_PW']) ) {
if ( $_SERVER['PHP_AUTH_USER'] === $username &&
$_SERVER['PHP_AUTH_PW'] === $password )
echo "You are now logged in";
else die("Invalid username/password combination");
} else {
header('WWW-Authenticate: Basic realm="Restricted Area"');
header('HTTP/1.0 401 Unauthorized');
die("Please enter your username and password");
}
?>